NumPy: Sum and compute the product of a NumPy array elements
Sum/Product of Array Elements
Write a NumPy program to sum and compute the product of a numpy array of elements.
Pictorial Presentation:
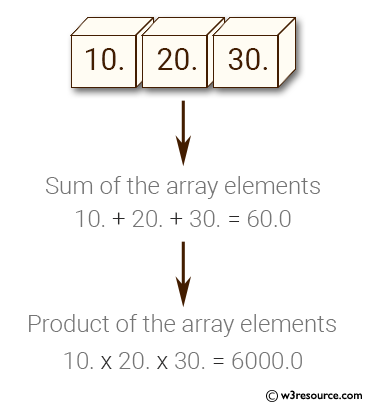
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'x' containing floating-point values
x = np.array([10, 20, 30], float)
# Printing a message indicating the original array will be displayed
print("Original array:")
# Printing the original array 'x' with its elements
print(x)
# Printing a message indicating the sum of the array elements will be displayed
print("Sum of the array elements:")
# Calculating and printing the sum of the elements in the array 'x' using 'sum()'
print(x.sum())
# Printing a message indicating the product of the array elements will be displayed
print("Product of the array elements:")
# Calculating and printing the product of the elements in the array 'x' using 'prod()'
print(x.prod())
Sample Output:
Original array: [ 10. 20. 30.] Sum of the array elements: 60.0 Product of the array elements: 6000.0
Explanation:
In the above exercise –
‘x = np.array([10, 20, 30], float)’ creates a NumPy array 'x' with the elements 10, 20, and 30 of float data type.
print(x.sum()): Calculate the sum of all elements in the 'x' array using the 'sum()' method and print the result. In this case, the sum is 10 + 20 + 30 = 60.
print(x.prod()): Calculate the product of all elements in the 'x' array using the 'prod()' method and print the result. In this case, the product is 10 * 20 * 30 = 6000.
For more Practice: Solve these Related Problems:
- Write a NumPy program to compute both the sum and product of all elements in an array using np.sum and np.prod.
- Create a function that returns a tuple with the sum and product of array elements, and validates the arithmetic operations.
- Test the sum and product operations on multi-dimensional arrays and verify that the calculations are independent of the axis.
- Compare the results of summing and multiplying elements manually with the outputs from NumPy’s built-in functions.
Go to:
PREV : Raw Array to Binary String/Back
NEXT : Use put to Place Values in Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.