Pandas SQL Query: Display the name, salary and department number for those employees whose first name ends with the letter 'm'
15. Display Employees Whose First Name Ends with 'm'
Write a Pandas program to display the first name, last name, salary and department number for those employees whose first name ends with the letter 'm'.
EMPLOYEES.csv
Sample Solution :
Python Code :
import pandas as pd
employees = pd.read_csv(r"EMPLOYEES.csv")
departments = pd.read_csv(r"DEPARTMENTS.csv")
job_history = pd.read_csv(r"JOB_HISTORY.csv")
jobs = pd.read_csv(r"JOBS.csv")
countries = pd.read_csv(r"COUNTRIES.csv")
regions = pd.read_csv(r"REGIONS.csv")
locations = pd.read_csv(r"LOCATIONS.csv")
print("First name Last name Salary Department ID")
result = employees[employees['first_name'].str[-1]=='m']
for index, row in result.iterrows():
print(row['first_name'].ljust(15),row['last_name'].ljust(15),str(row['salary']).ljust(9),row['department_id'])
Sample Output:
First name Last name Salary Department ID Adam Fripp 8200 50.0 Payam Kaufling 7900 50.0 William Smith 7400 80.0 William Gietz 8300 110.0
Click to view the table contain:
For more Practice: Solve these Related Problems:
- Write a Pandas program to display the first name, last name, salary, and department number for employees whose first name ends with 'm' using regex.
- Write a Pandas program to perform a case-insensitive search for employees whose first name ends with 'm' and display the results.
- Write a Pandas program to filter employees with first names ending in 'm', then sort the results by salary.
- Write a Pandas program to display the required fields for employees with first names ending with 'm' and check for any null values in the first name.
Go to:
Previous: Write a Pandas program to count the NaN values of all the columns of locations file.
Next: Write a Pandas program to display the first name, last name, salary and department number for those employees whose first name ends with the letter 'd' or 'n' or 's' and also arrange the result in descending order by department id.
Python Code Editor:
Structure of HR database :
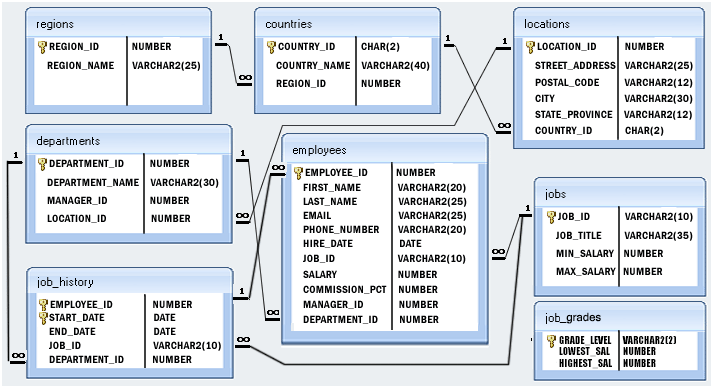
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?