Sorting Pandas DataFrame by values: Python data manipulation
Python Pandas Numpy: Exercise-28 with Solution
Sort a Pandas DataFrame by values in a specific column.
Sample Solution:
Python Code:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['Imen', 'Karthika', 'Cosimo', 'Cathrine'],
'Age': [25, 30, 22, 35],
'Salary': [50000, 60000, 45000, 70000]}
df = pd.DataFrame(data)
# Sort the DataFrame by the 'Salary' column in ascending order
sorted_df = df.sort_values(by='Salary')
# Display the sorted DataFrame
print(sorted_df)
Output:
Name Age Salary 2 Cosimo 22 45000 0 Imen 25 50000 1 Karthika 30 60000 3 Cathrine 35 70000
Explanation:
Here's a breakdown of the above code:
- We created a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- The df.sort_values(by='Salary') method sorts the DataFrame based on the values in the 'Salary' column in ascending order.
- The resulting "sorted_df" DataFrame is displayed, showing the rows sorted by the 'Salary' column.
Flowchart:
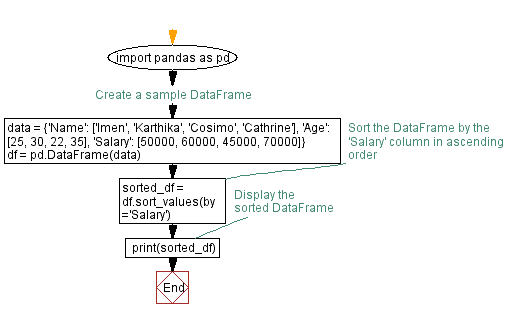
Python Code Editor:
Previous: Generating and identifying unique values in a NumPy array.
Next: Applying custom function to salary: Pandas DataFrame operation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics