Selecting rows based on multiple conditions in Pandas DataFrame
Select rows from a DataFrame based on multiple conditions.
Sample Solution:
Python Code:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['Teodosija', 'Sutton', 'Taneli', 'Ravshan', 'Ross'],
'Age': [26, 32, 25, 31, 28],
'Salary': [50000, 60000, 45000, 70000, 55000]}
df = pd.DataFrame(data)
# Select rows based on multiple conditions
selected_rows = df[(df['Age'] > 25) & (df['Salary'] > 50000)]
# Display the selected rows
print(selected_rows)
Output:
Name Age Salary 1 Sutton 32 60000 3 Ravshan 31 70000 4 Ross 28 55000
Explanation:
- Importing Pandas:
import pandas as pd
Imports the Pandas library and aliases it as "pd" for convenience. - Creating a Sample DataFrame:
data = {'Name': ['Teodosija', 'Sutton', 'Taneli', 'Ravshan', 'Ross'], 'Age': [26, 32, 25, 31, 28], 'Salary': [50000, 60000, 45000, 70000, 55000]} df = pd.DataFrame(data)
Creates a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'. - Selecting Rows Based on Multiple Conditions:
selected_rows = df[(df['Age'] > 25) & (df['Salary'] > 50000)]
Uses boolean indexing to select rows where both conditions are true: age is greater than 25 and salary is greater than 50000. - Displaying the Selected Rows:
print(selected_rows)
Prints the selected rows to the console.
Flowchart:
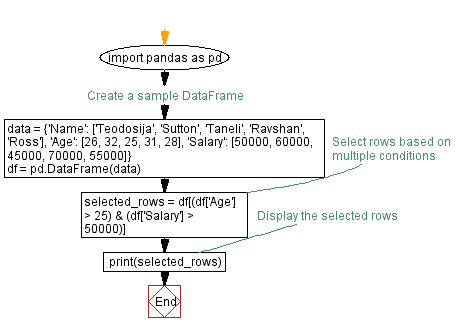
Python Code Editor:
Previous: Generating a Pandas DataFrame from a NumPy array with custom column names in Python.
Next: Selecting the first and last 7 rows in a Pandas DataFrame.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.