Merging DataFrames based on a common column in Pandas
Python Pandas Numpy: Exercise-7 with Solution
Merge two Pandas DataFrames based on a common column.
Sample Solution:
Python Code:
import pandas as pd
# Create two sample DataFrames
df1 = pd.DataFrame({'ID': [1, 2, 3], 'Name': ['Teodosija', 'Sutton', 'Taneli']})
df2 = pd.DataFrame({'ID': [2, 3, 4], 'Age': [25, 30, 22]})
# Merge DataFrames based on the 'ID' column
merged_df = pd.merge(df1, df2, on='ID')
# Display the merged DataFrame
print(merged_df)
Output:
ID Name Age 0 2 Sutton 25 1 3 Taneli 30
Explanation:
In the exerciser above -
- First we create two sample DataFrames, "df1" and "df2", with a common column 'ID'.
- The pd.merge function is used to merge the DataFrames based on the 'ID' column.
- The on='ID' parameter specifies the common column on which the merge operation is performed.
- The resulting DataFrame (merged_df) contains columns from both DataFrames, and rows are matched based on the values in the 'ID' column.
Flowchart:
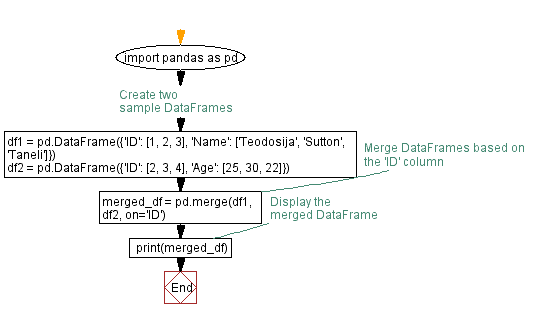
Python Code Editor:
Previous: Merging DataFrames based on a common column in Pandas.
Next: Filtering DataFrame rows by column values in Pandas using NumPy array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pandas_numpy/pandas_numpy-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics