Python: Test whether the given strings are palindromes
Check Palindromes in List
Write a Python program to check whether the given strings are palindromes or not. Return True otherwise False.
Input: ['palindrome', 'madamimadam', '', 'foo', 'eyes'] Output: [False, True, True, False, False]
Visual Presentation:
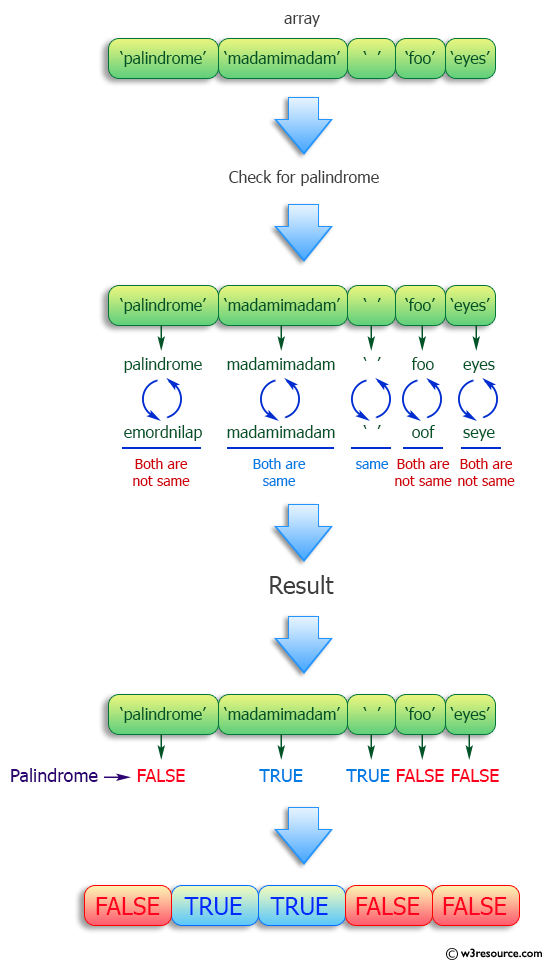
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'strs' as input
def test(strs):
# Use a list comprehension to check if each string in 'strs' is a palindrome (reads the same forwards and backwards)
return [s == s[::-1] for s in strs]
# Create a list of strings 'strs' with specific elements
strs = ['palindrome', 'madamimadam', '', 'foo', 'eyes']
# Print the original list of strings
print("Original strings:")
print(strs)
# Print a message indicating the operation to be performed on the list
print("\nTest whether the given strings are palindromes or not:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
Sample Output:
Original strings: ['palindrome', 'madamimadam', '', 'foo', 'eyes'] Test whether the given strings are palindromes or not: [False, True, True, False, False]
Flowchart:
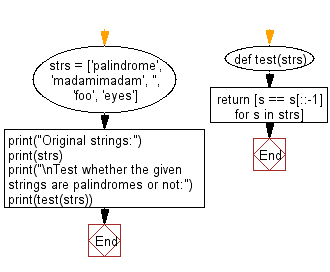
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes a list of strings 'strs' as input
def test(strs):
# Initialize an empty list 'results' to store the results of palindrome tests
results = []
# Iterate through each string in 'strs'
for _ in strs:
# Padding each string with spaces on both sides
# By default, the pad_to parameter is 50
s = ' ' * 50 + _ + ' ' * 50
# Initialize variables 'i' and 'j' for indexing the string 's'
i = 0
j = len(s) - 1
# Use a while loop to check if the string 's' is a palindrome
while i < j:
if s[i] != s[j]:
# If characters at positions 'i' and 'j' are not equal, append False to 'results' and break out of the loop
results.append(False)
break
else:
# Increment 'i' and decrement 'j' to compare the next pair of characters
i += 1
j -= 1
else:
# If the while loop completes without a break, append True to 'results'
results.append(True)
# Return the list of results
return results
# Create a list of strings 'strs' with specific elements
strs = ['palindrome', 'madamimadam', '', 'foo', 'eyes']
# Print the original list of strings
print("Original strings:")
print(strs)
# Print a message indicating the operation to be performed on the list
print("\nTest whether the given strings are palindromes or not:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
Sample Output:
Original strings: ['palindrome', 'madamimadam', '', 'foo', 'eyes'] Test whether the given strings are palindromes or not: [False, True, True, False, False]
Flowchart:
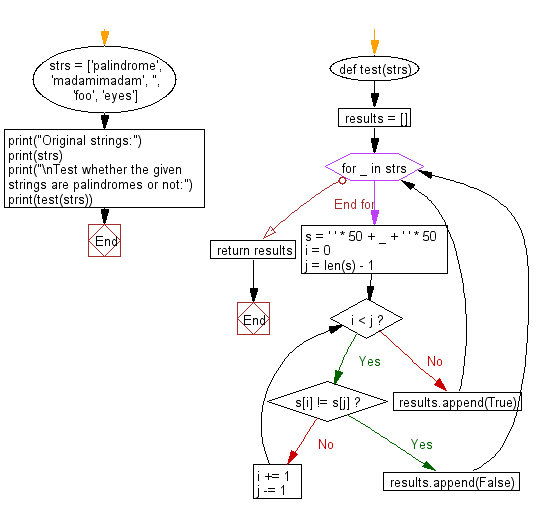
For more Practice: Solve these Related Problems:
- Write a Python program to check each string in a list and return a list of boolean values indicating whether each string is a palindrome.
- Write a Python program to filter a list of strings and output only those that read the same forwards and backwards.
- Write a Python program to verify palindrome properties for each string using slicing and list comprehension.
- Write a Python program to implement a helper function that checks for palindromes and map it over a list of strings.
Go to:
Previous: Find the indexes of numbers, below a given threshold.
Next: Find the strings in a list, starting with a given prefix.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.