Python: Find the largest number where commas or periods are decimal points
Largest Number with Commas/Periods
Write a Python program to find the largest number where commas or periods are decimal points.
Input: ['100', '102,1', '101.1'] Output: 102.1
Visual Presentation:
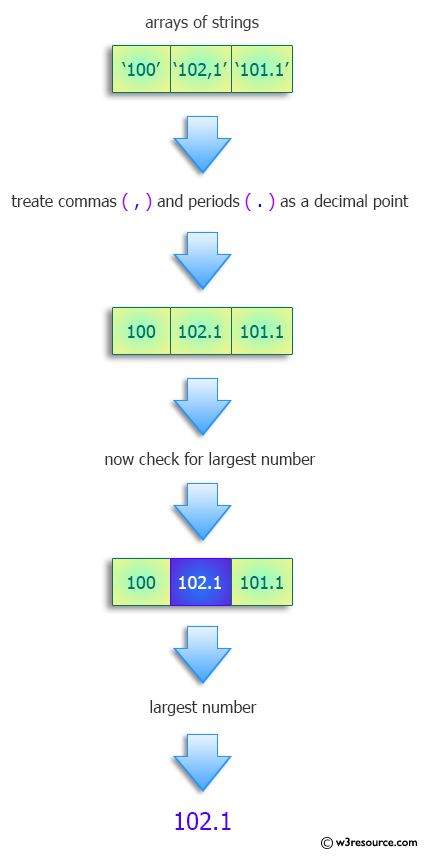
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'str_nums' as input
def test(str_nums):
# Use a generator expression to iterate through each string in 'str_nums'
# Replace commas with periods and convert each string to a float, then find the maximum value
return max(float(s.replace(",", ".")) for s in str_nums)
# Assign a specific list of strings 'str_nums' to the variable
str_nums = ["100", "102,1", "101.1"]
# Print the original list of strings 'str_nums'
print("Original list:")
print(str_nums)
# Print a message indicating the operation to be performed
print("Largest number where commas or periods are decimal points:")
# Print the result of the test function applied to the 'str_nums' list
print(test(str_nums))
Sample Output:
Original list: ['100', '102,1', '101.1'] Largest number where commas or periods are decimal points: 102.1
Flowchart:
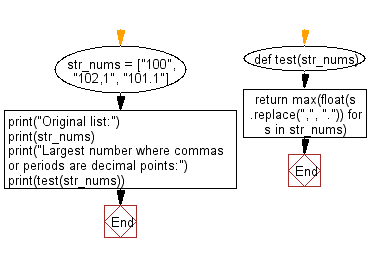
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'str_nums' as input
def test(str_nums):
# Initialize an empty list to store converted float values
numbers = []
# Iterate through each string in 'str_nums'
for s in str_nums:
# Replace commas with periods, convert the string to a float, and append to the 'numbers' list
numbers.append(float(s.replace(",", ".")))
# Sort the 'numbers' list in ascending order
numbers.sort()
# Return the largest number, which is the last element in the sorted list
return numbers[-1]
# Assign a specific list of strings 'str_nums' to the variable
str_nums = ["100", "102,1", "103.1"]
# Print the original list of strings 'str_nums'
print("Original list:")
print(str_nums)
# Print a message indicating the operation to be performed
print("Largest number where commas or periods are decimal points:")
# Print the result of the test function applied to the 'str_nums' list
print(test(str_nums))
Sample Output:
Original list: ['100', '102,1', '103.1'] Largest number where commas or periods are decimal points: 103.1
Flowchart:
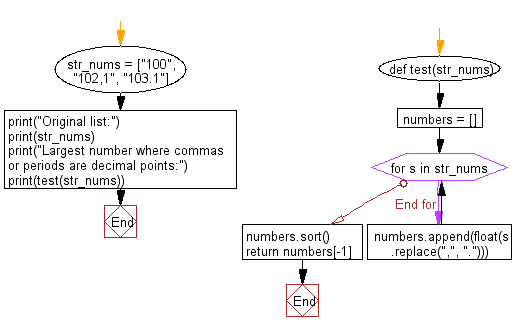
For more Practice: Solve these Related Problems:
- Write a Python program to parse a list of number strings where commas and periods are used as decimal points and determine the largest value.
- Write a Python program to convert strings with mixed decimal separators into floats and find the maximum.
- Write a Python program to normalize number strings with commas or periods as decimals and output the highest numerical value.
- Write a Python program to use regular expressions to extract numbers from strings with varying decimal delimiters and determine the largest one.
Go to:
Previous: Find the XOR of two given strings interpreted as binary numbers.
Next: Find x that minimizes mean squared deviation from a given list of numbers.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.