Python: Find the positions of all uppercase vowels (not counting Y) in even indices
Python Programming Puzzles: Exercise-33 with Solution
Uppercase Vowel Positions
Write a Python program to find the positions of all uppercase vowels (not counting Y) in even indices of a given string.
From Wikipedia:
A vowel is a syllabic speech sound pronounced without any stricture in the vocal tract. Vowels are one of the two principal classes of speech sounds, the other being the consonant. Vowels vary in quality, in loudness and also in quantity. There are six vowels in the English language: a, e, i, o, u and sometimes y.
Input: w3rEsOUrcE Output: [6] Input: AEIOUYW Output: [0, 2, 4]
Visual Presentation:
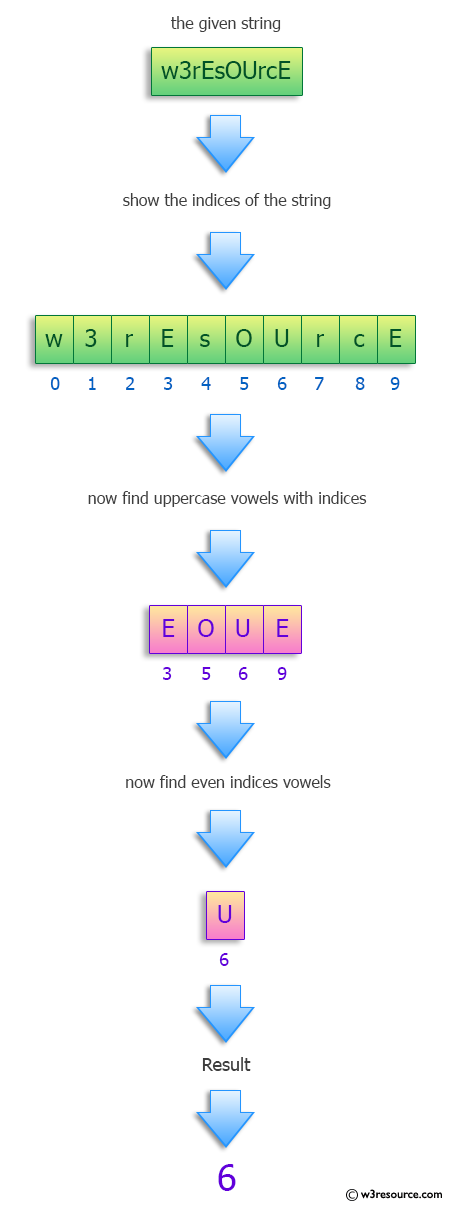
Sample Solution:
Python Code:
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Use a list comprehension to generate a list of indices for uppercase vowels (excluding 'Y') at even indices
return [i for i, c in enumerate(strs) if i % 2 == 0 and c in "AEIOU"]
# Assign a specific string 'strs' to the variable
strs = "w3rEsOUrcE "
# Print a message indicating the operation to be performed
print("Original List:", strs)
# Print the original string
print("Positions of all uppercase vowels (not counting Y) in even indices:")
# Print the result of the test function applied to the 'strs' string
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "AEIOUYW "
# Print a message indicating the operation to be performed
print("\nOriginal List:", strs)
# Print the original string
print("Positions of all uppercase vowels (not counting Y) in even indices:")
# Print the result of the test function applied to the 'strs' string
print(test(strs))
Sample Output:
Original List: w3rEsOUrcE Positions of all uppercase vowels (not counting Y) in even indices: [6] Original List: AEIOUYW Positions of all uppercase vowels (not counting Y) in even indices: [0, 2, 4]
Flowchart:
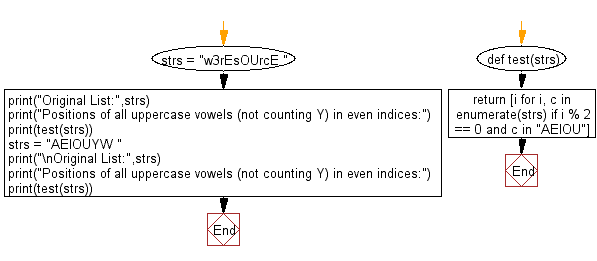
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Rescale and shift numbers so that they cover the range [0, 1].
Next: Find the sum of the numbers among the first k with more than 2 digits.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/puzzles/python-programming-puzzles-33.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics