Python: Sum of the magnitudes of the elements in the array with product signs
Python Programming Puzzles: Exercise-57 with Solution
Signed Sum of Magnitudes
Write a Python program to find the sum of the magnitudes of the elements in the array. This sum should have a sign that is equal to the product of the signs of the entries.
Input: [1, 3, -2] Output: -6 Input: [1, -3, 3] Output: -7 Input: [10, 32, 3] Output: 45 Input: [-25, -12, -23] Output: -60
Visual Presentation:
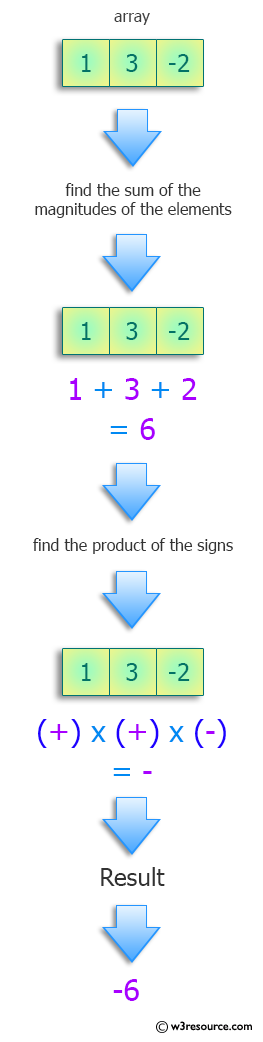
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers as a parameter
def test(nums):
# Calculate the total sum of the magnitudes of the elements in the array
tot = sum(abs(i) for i in nums)
# Check if all elements in the array are non-zero
if all(nums):
# Return the total sum with a positive or negative sign based on the product of the signs of the entries
return tot if sum(i < 0 for i in nums) % 2 == 0 else -tot
# If there is a zero element in the array, return 0
return 0
# Set a list of numbers as the input for the test function
nums = [1, 3, -2]
# Print a message indicating the original list of numbers
print("Original list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the task and the result of the test function
print("Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries:")
# Print the result of the test function applied to the given list of numbers
print(test(nums))
# Set a new list of numbers as the input for the test function
nums = [1, -3, 3]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the task and the result of the test function
print("Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries:")
# Print the result of the test function applied to the new list of numbers
print(test(nums))
# Set another new list of numbers as the input for the test function
nums = [10, 32, 3]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the task and the result of the test function
print("Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries:")
# Print the result of the test function applied to the new list of numbers
print(test(nums))
# Set yet another new list of numbers as the input for the test function
nums = [-25, -12, -23]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the task and the result of the test function
print("Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries:")
# Print the result of the test function applied to the new list of numbers
print(test(nums))
Sample Output:
Original list of numbers: [1, 3, -2] Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries: -6 Original list of numbers: [1, -3, 3] Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries: -7 Original list of numbers: [10, 32, 3] Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries: 45 Original list of numbers: [-25, -12, -23] Sum of the magnitudes of the elements in the array with a sign that is equal to the product of the signs of the entries: -60
Flowchart:
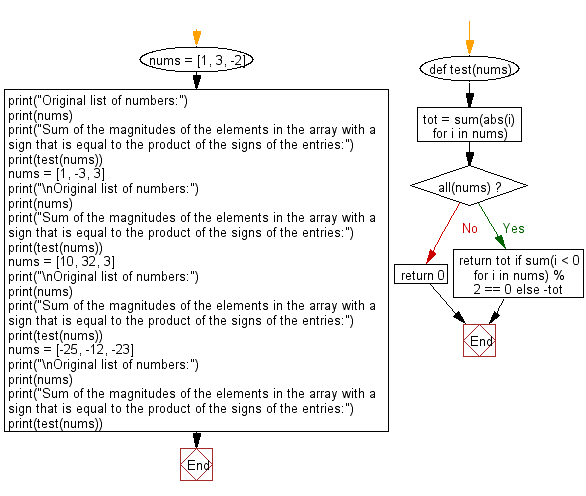
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find an integer exponent x such that a^x = n.
Next: Biggest even number between two numbers inclusive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/puzzles/python-programming-puzzles-57.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics