Python: Find a string contains a vowel between two consonants, in a given string
Python Programming Puzzles: Exercise-73 with Solution
Write a Python program to find a substring in a given string that contains a vowel between two consonants.
From vocabulary.com:
A consonant is a speech sound that is not a vowel. It also refers to letters of the alphabet that represent those sounds: Z, B, T, G, and H are all consonants. Consonants are all the non-vowel sounds, or their corresponding letters: A, E, I, O, U and sometimes Y are not consonants.
Input: Hello Output: Hel Input: Sandwhich Output: San Input: Python Output: hon
Visual Presentation:
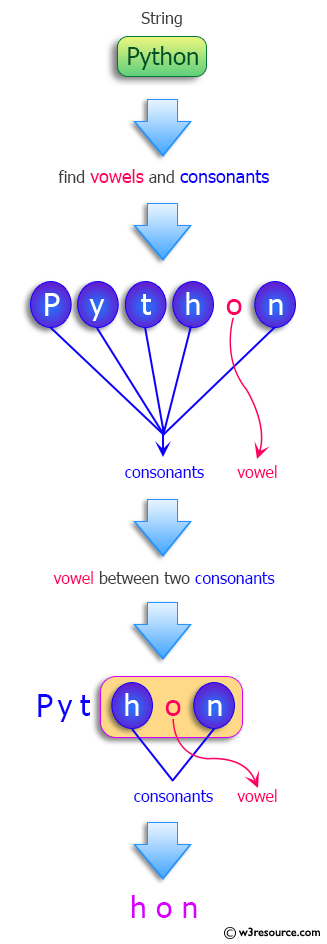
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string as input
def test(s):
cons = "bcdfghjklmnpqrstvwxz" # Define a string containing consonants
vows = "aeiou" # Define a string containing vowels
# Use a generator expression to find a vowel between two consonants in the string
return next(s[i - 1:i + 2] for i in range(1, len(s) - 1)
if s[i].lower() in vows and s[i - 1].lower() in cons and s[i + 1].lower() in cons)
# Example 1
strs1 = "Hello"
print("Original string:", strs1)
print("Find a vowel between two consonants, contained in said string:")
print(test(strs1))
# Example 2
strs2 = "Sandwich"
print("\nOriginal string:", strs2)
print("Find a vowel between two consonants, contained in said string:")
print(test(strs2))
# Example 3
strs3 = "Python"
print("\nOriginal string:", strs3)
print("Find a vowel between two consonants, contained in said string:")
print(test(strs3))
Sample Output:
Original string: Hello Find a vowel between two consonants, contained in said string: Hel Original string: Sandwhich Find a vowel between two consonants, contained in said string: San Original string: Python Find a vowel between two consonants, contained in said string: hon
Flowchart:
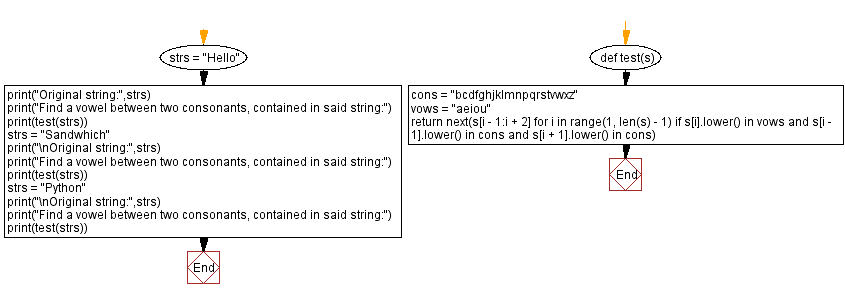
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the indices of three numbers that sum to 0 in a list.
Next: Find a string consisting of space-separated characters with given counts.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics