Python: Reorder numbers in increasing/decreasing order based on whether the first plus last element is even/odd
Reorder Numbers Based on Sum
Write a Python program to reorder numbers from a given array in increasing/decreasing order based on whether the first plus last element is odd/even.
Input: [3, 7, 4] Output: [3, 4, 7] Input: [2, 7, 4] Output: [7, 4, 2] Input: [1, 5, 6, 7, 4, 2, 8] Output: [1, 2, 4, 5, 6, 7, 8] Input: [1, 5, 6, 7, 4, 2, 9] Output: [9, 7, 6, 5, 4, 2, 1]
Visual Presentation:
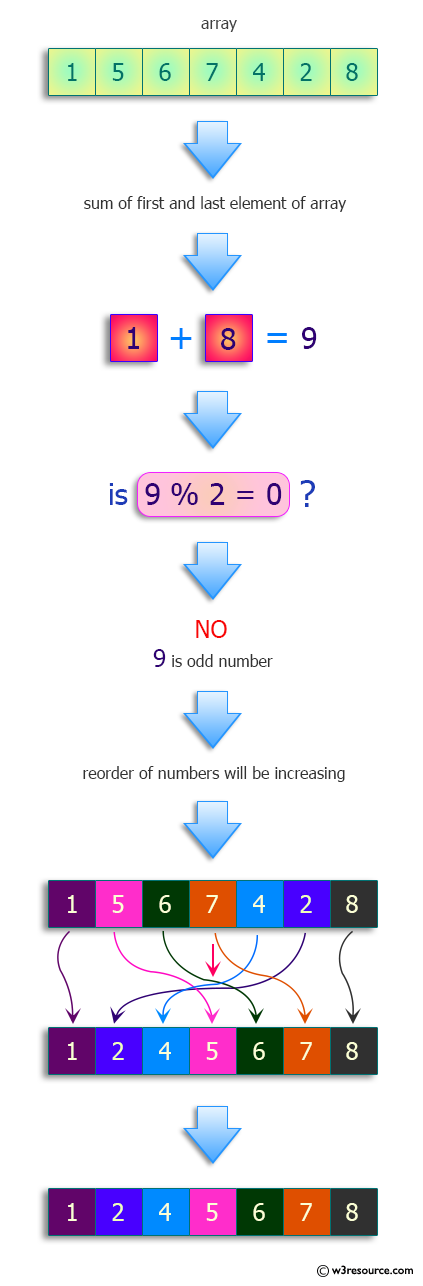
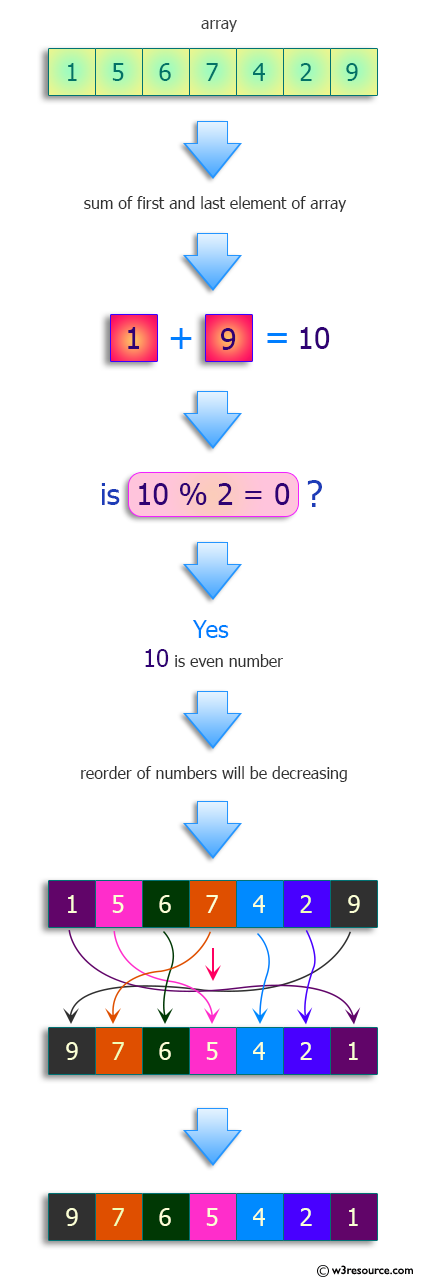
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
# Use the sorted function to reorder the numbers based on whether the sum of the first and last element is odd/even
return sorted(nums, reverse=(False if (nums[0] + nums[-1]) % 2 else True))
# Print a message indicating the purpose of the code
print("Reorder numbers of a given array in increasing/decreasing order based on whether the first plus last element is odd/even.")
# Example 1
nums1 = [3, 7, 4]
print("\nList of numbers:", nums1)
print("Result:")
print(test(nums1))
# Example 2
nums2 = [2, 7, 4]
print("\nList of numbers:", nums2)
print("Result:")
print(test(nums2))
# Example 3
nums3 = [1, 5, 6, 7, 4, 2, 8]
print("\nList of numbers:", nums3)
print("Result:")
print(test(nums3))
# Example 4
nums4 = [1, 5, 6, 7, 4, 2, 9]
print("\nList of numbers:", nums4)
print("Result:")
print(test(nums4))
Sample Output:
Reorder numbers of a give array in increasing/decreasing order based on whether the first plus last element is odd/even.: List of numbers: [3, 7, 4] Result: [3, 4, 7] List of numbers: [2, 7, 4] Result: [7, 4, 2] List of numbers: [1, 5, 6, 7, 4, 2, 8] Result: [1, 2, 4, 5, 6, 7, 8] List of numbers: [1, 5, 6, 7, 4, 2, 9] Result: [9, 7, 6, 5, 4, 2, 1]
Flowchart:
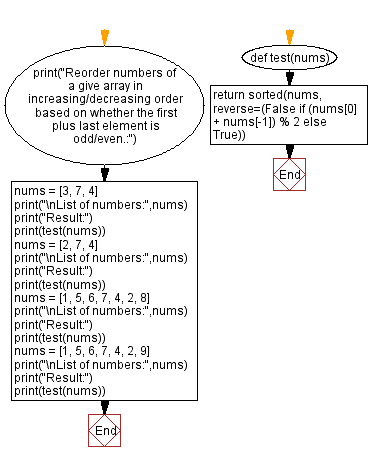
For more Practice: Solve these Related Problems:
- Write a Python program to sort a list in increasing order if the sum of the first and last elements is odd, otherwise in decreasing order.
- Write a Python program to reorder an array by checking the parity of the sum of its first and last elements and sorting accordingly.
- Write a Python program to use conditional logic to sort a list ascendingly or descendingly based on whether the first plus last element is odd or even.
- Write a Python program to implement a function that sorts the list in two different ways determined by the sum of its outer elements.
Go to:
Previous: Find a string consisting of space-separated characters with given counts.
Next: Find the index of the largest prime in the list and the sum of its digits.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.