Python: Find the largest negative and smallest positive numbers
Largest Negative and Smallest Positive Numbers
Write a Python program to find the largest negative and smallest positive numbers (or 0 if none).
Input: [-12, -6, 300, -40, 2, 2, 3, 57, -50, -22, 12, 40, 9, 11, 18] Output: [-6, 2] Input: [-1, -2, -3, -4] Output: [-1, 0] Input: [1, 2, 3, 4] Output: [0, 1] Input: [] Output: [0, 0]
Visual Presentation:
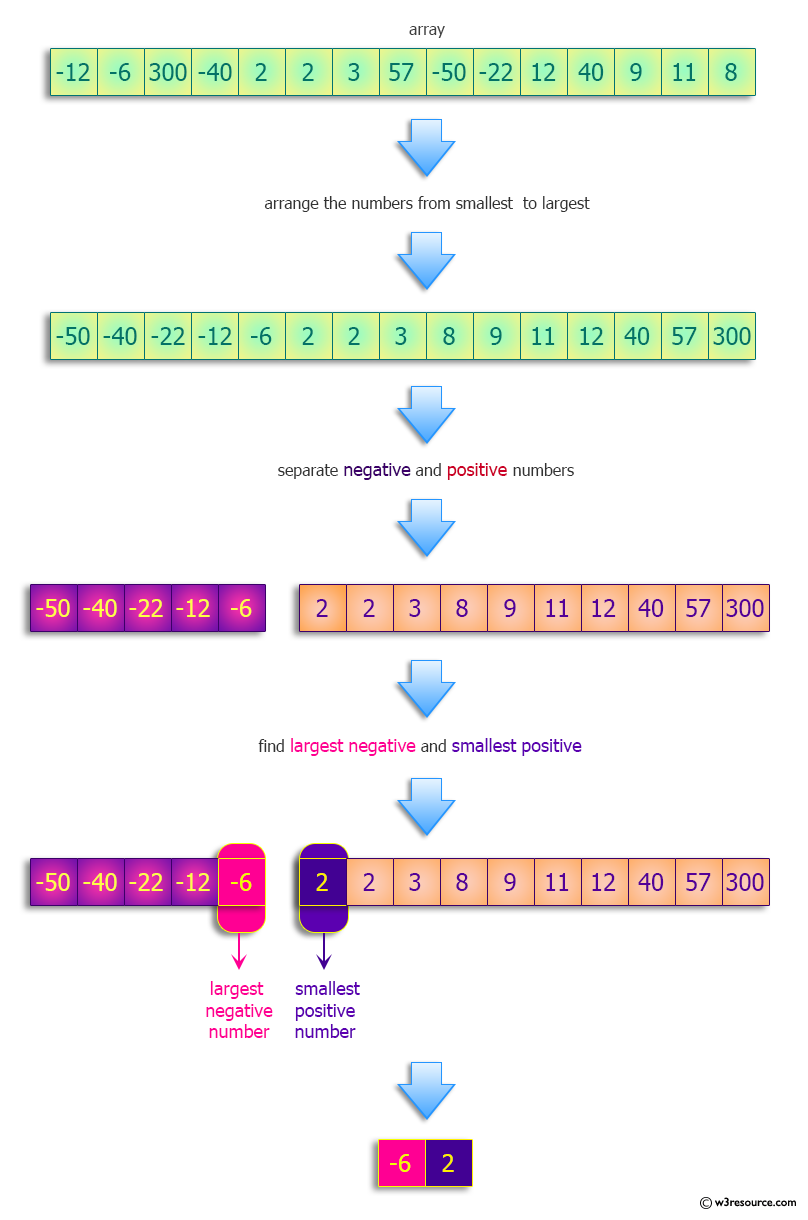
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
# Create a list 'pos' containing positive numbers from the input list
pos = [n for n in nums if n > 0]
# Create a list 'neg' containing negative numbers from the input list
neg = [n for n in nums if n < 0]
# Return a list containing the largest negative number (or 0 if none) and the smallest positive number (or 0 if none)
return [max(neg) if neg else 0, min(pos) if pos else 0]
# Example 1
nums1 = [-12, -6, 300, -40, 2, 2, 3, 57, -50, -22, 12, 40, 9, 11, 18]
print("List of numbers:", nums1)
print("Largest negative and smallest positive numbers (or 0 if none) of the said list:")
print(test(nums1))
# Example 2
nums2 = [-1, -2, -3, -4]
print("\nList of numbers:", nums2)
print("Largest negative and smallest positive numbers (or 0 if none) of the said list:")
print(test(nums2))
# Example 3
nums3 = [1, 2, 3, 4]
print("\nList of numbers:", nums3)
print("Largest negative and smallest positive numbers (or 0 if none) of the said list:")
print(test(nums3))
# Example 4
nums4 = []
print("\nList of numbers:", nums4)
print("Largest negative and smallest positive numbers (or 0 if none) of the said list:")
print(test(nums4))
Sample Output:
List of numbers: [-12, -6, 300, -40, 2, 2, 3, 57, -50, -22, 12, 40, 9, 11, 18] Largest negative and smallest positive numbers (or 0 if none) of the said list: [-6, 2] List of numbers: [-1, -2, -3, -4] Largest negative and smallest positive numbers (or 0 if none) of the said list: [-1, 0] List of numbers: [1, 2, 3, 4] Largest negative and smallest positive numbers (or 0 if none) of the said list: [0, 1] List of numbers: [] Largest negative and smallest positive numbers (or 0 if none) of the said list: [0, 0]
Flowchart:
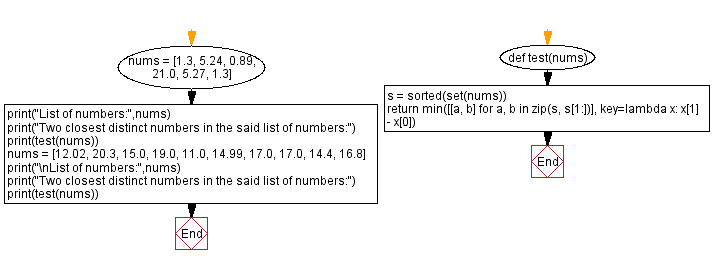
For more Practice: Solve these Related Problems:
- Write a Python program to scan a list and return the largest negative and smallest positive numbers, using conditional filters.
- Write a Python program to separate negatives and positives from a list, then output the maximum negative and minimum positive.
- Write a Python program to use list comprehensions to extract negative and positive numbers and then select the required values.
- Write a Python program to implement a single-pass algorithm that determines the largest negative and smallest positive numbers.
Go to:
Previous: Find the two closest distinct numbers in a given a list of numbers.
Next: Round each float in a list of numbers up to the next integer and return the running total of the integer squares.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.