Python PyQt checkbox example
Python PyQt Connecting Signals to Slots: Exercise-13 with Solution
Write a Python program that creates a window with a checkbox, like a "yes" or "no" choice. When you click the checkbox to check or uncheck it, something should happen. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QCheckBox Class: The QCheckBox widget provides a checkbox with a text label.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QLabel Class: The QLabel widget provides a text or image display.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QCheckBox, QVBoxLayout, QLabel
class CheckBoxApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Checkbox Example")
self.setGeometry(100, 100, 300, 100) # (x, y, width, height)
layout = QVBoxLayout()
# Create a checkbox
self.checkbox = QCheckBox("Check me", self)
layout.addWidget(self.checkbox)
# Create a label to display the checkbox state
self.checkbox_state_label = QLabel("Checkbox state: Unchecked", self)
layout.addWidget(self.checkbox_state_label)
# Connect the checkbox's stateChanged signal to a slot that reacts to changes
self.checkbox.stateChanged.connect(self.update_checkbox_state)
self.setLayout(layout)
def update_checkbox_state(self):
# Check the state of the checkbox and update the label accordingly
if self.checkbox.isChecked():
self.checkbox_state_label.setText("Checkbox state: Checked")
else:
self.checkbox_state_label.setText("Checkbox state: Unchecked")
def main():
app = QApplication(sys.argv)
window = CheckBoxApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QWidget" class named "CheckBoxApp" that builds a window with a checkbox.
- Inside the window, there's a checkbox (self.checkbox) and a label (self.checkbox_state_label).
- Connect the checkbox's stateChanged signal to a slot function (self.update_checkbox_state) that reacts to changes in the checkbox's state.
- When you check or uncheck the checkbox, the label displays whether it's checked or unchecked.
- In the main function, we create the PyQt application, instantiate the "CheckBoxApp" class, and show the main window.
Output:
Flowchart:
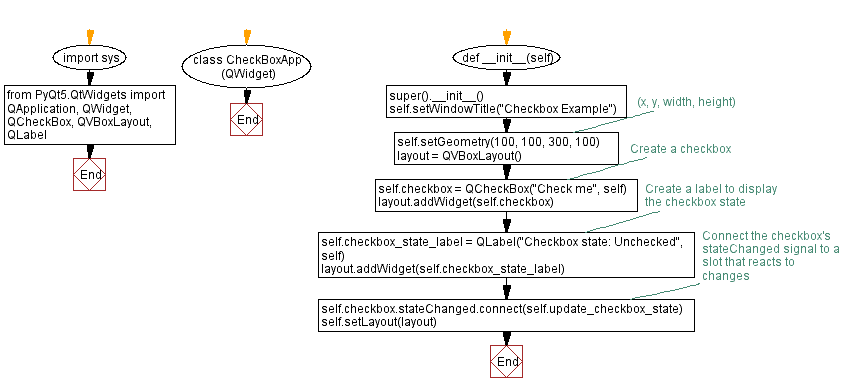
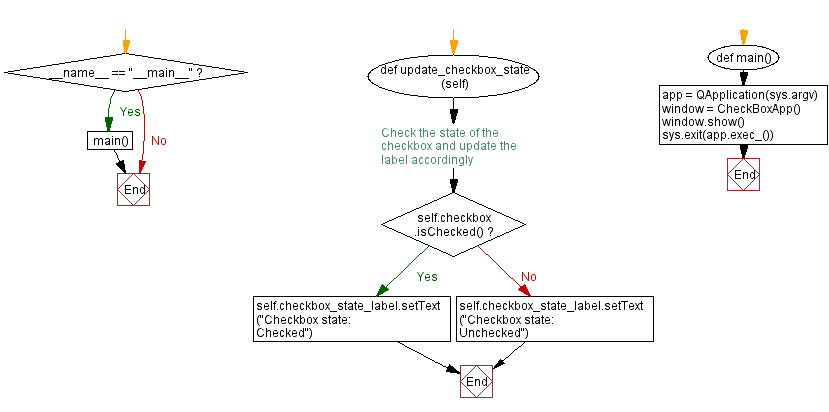
Python Code Editor:
Previous: Python PyQt time display.
Next: Python PyQt menu example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pyqt/python-pyqt-connecting-signals-to-slots-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics