Python: Count vowels in python using function
Python Basic: Exercise-24 with Solution
Write a Python program to test whether a passed letter is a vowel or not.
Pictorial Presentation:
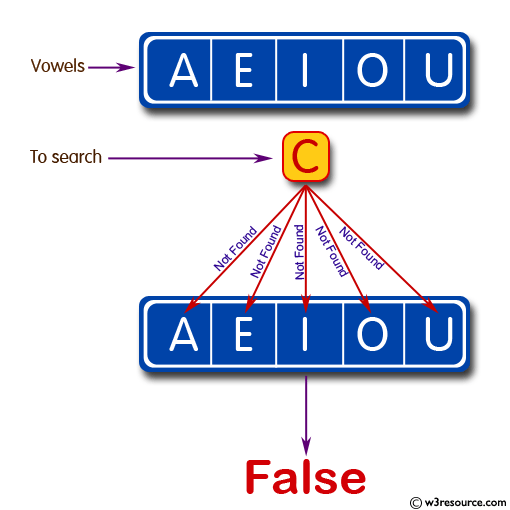
Sample Solution:
Python Code:
# Define a function called is_vowel that takes one parameter: char (a character).
def is_vowel(char):
# Define a string called all_vowels containing all lowercase vowel characters.
all_vowels = 'aeiou'
# Check if the input character (char) is present in the all_vowels string.
return char in all_vowels
# Call the is_vowel function with two different characters and print the results.
print(is_vowel('c')) # Output: False (character 'c' is not a vowel)
print(is_vowel('e')) # Output: True (character 'e' is a vowel)
Sample Output:
False True
Explanation:
The said code defines a function called "is_vowel" which takes in one parameter "char". First, the function declares a variable "all_vowels" containing all lowercase vowels. The function then uses the "in" operator to check whether the value of "char" parameter is present within the "all_vowels" string, and returns the result.
In the last two lines the code call the function "is_vowel" twice, with the input values 'c' and 'e' and print the results.
In the first case, False will be returned, while in the second case, True will be returned.
Flowchart:
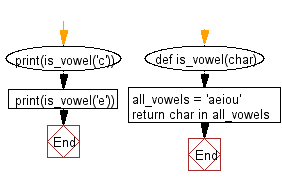
Python Code Editor:
Previous: Write a Python program to get the n (non-negative integer) copies of the first 2 characters of a given string. Return the n copies of the whole string if the length is less than 2.
Next: Write a Python program to check whether a specified value is contained in a group of values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics