Python: Return true if the two given integer values are equal or their sum or difference is 5
Equality or 5 Rule Checker
Write a Python program that returns true if the two given integer values are equal or their sum or difference is 5.
Pictorial Presentation:
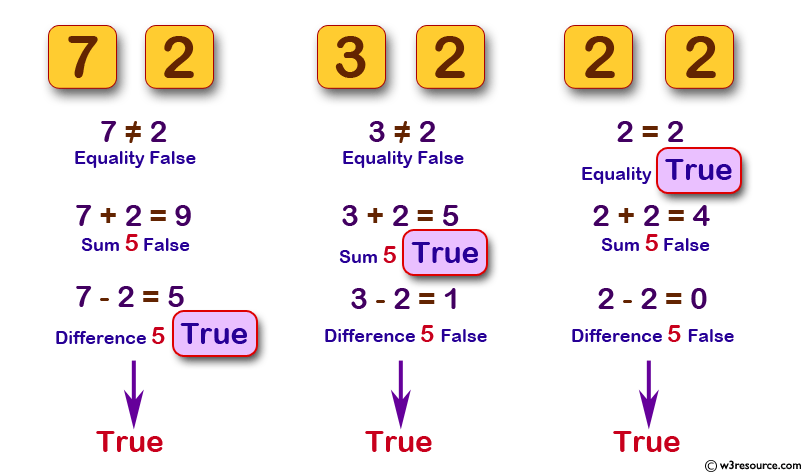
Sample Solution:
Python Code:
# Define a function 'test_number5' that takes two integer inputs: x and y.
def test_number5(x, y):
# Check if any of the following conditions are met:
# 1. x is equal to y.
# 2. The absolute difference between x and y is equal to 5.
# 3. The sum of x and y is equal to 5.
if x == y or abs(x - y) == 5 or (x + y) == 5:
# If any of the conditions are met, return True.
return True
else:
# If none of the conditions are met, return False.
return False
# Test the 'test_number5' function with different sets of input values and print the results.
print(test_number5(7, 2))
print(test_number5(3, 2))
print(test_number5(2, 2))
print(test_number5(7, 3))
print(test_number5(27, 53))
Sample Output:
True True True False False
Explanation:
The said code defines a function "test_number5(x, y)" that takes two integers "x" and "y" as arguments and check if they are equal, or if their sum or difference is equal to 5. If any of these conditions is true, the program would return "True", otherwise, it would return "False".
At the end, the function is called five times with different inputs and prints the outputs of the inputs.
Flowchart:
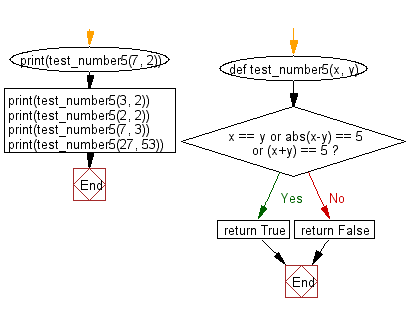
For more Practice: Solve these Related Problems:
- Write a Python program that returns True if two numbers are equal, their sum is 10, or their absolute difference is 3.
- Write a function that returns True if two given numbers are both odd or both even.
- Write a script that checks if two numbers are multiples of each other or their sum is a multiple of 5.
- Write a Python program that returns True if either of two numbers is prime, or their sum is a prime number.
Go to:
Previous: Write a Python program to sum of two given integers. However, if the sum is between 15 to 20 it will return 20.
Next: Write a Python program to add two objects if both objects are an integer type.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.