Python: List all files in a directory in Python
Python Basic: Exercise-49 with Solution
Write a Python program to list all files in a directory.
Sample Solution:-
Python Code:
# Import necessary functions and modules from the 'os' library.
from os import listdir
from os.path import isfile, join
# Create a list 'files_list' that contains the names of files in the '/home/students' directory.
# It uses a list comprehension to filter files using 'isfile' and 'join' functions.
files_list = [f for f in listdir('/home/students') if isfile(join('/home/students', f))]
# Print the list of file names.
print(files_list)
Sample Output:
['3ff44d80-2423-11e7-807b-bd9de91b1602.py', 'f5272c90-2423-11e7-807b-bd9de91b1602.py', 'test.txt', 'f34e26d0-2 423-11e7-807b-bd9de91b1602.py', 'f6a62b70-2423-11e7-807b-bd9de91b1602.py', '.mysql_history', '.bash_logout', ' 037db660-240b-11e7-807b-bd9de91b1602.py', '3d299190-241f-11e7-807b-bd9de91b1602.py', '8e7e22f0-240f-11e7-807b- bd9de91b1602.py', '.bash_history', '276b1dd0-2404-11e7-807b-bd9de91b1602.py', '7e2f05d0-241a-11e7-807b-bd9de91 b1602.py', '6956ba40-2429-11e7-807b-bd9de91b1602.py', '.profile', 'abc.py', 'f69a4490-2423-11e7-807b-bd9de91b1 602.py', '.viminfo', 'mynewtest.txt', 'myfile.txt', 'logging_example.out', '.web-term.json', 'd2c942f0-2423-11 e7-807b-bd9de91b1602.py', 'ca7c2750-242a-11e7-807b-bd9de91b1602.py', 'abc.txt', 'exercise.cs', '.bashrc', 'Exa mple.cs', '2f5aa190-2428-11e7-807b-bd9de91b1602.py', '2d5d6540-2418-11e7-807b-bd9de91b1602.py', '5bfc84e0-240d -11e7-807b-bd9de91b1602.py', '940ca5b0-2406-11e7-807b-bd9de91b1602.py', 'myfig.png', 'file.out', 'a7af6660-241 6-11e7-807b-bd9de91b1602.py', '4a690da0-2428-11e7-807b-bd9de91b1602.py', 'line.gif', 'mmm.txt\n', 'temp.txt', 'fb6e28e0-2425-11e7-807b-bd9de91b1602.py', 'f0896180-2423-11e7-807b-bd9de91b1602.py', 'dddd.txt\n', '700da350- 2413-11e7-807b-bd9de91b1602.py', 'sss.dat\n', '05f2fa20-2421-11e7-807b-bd9de91b1602.py', 'result.txt', '68c38d e0-241c-11e7-807b-bd9de91b1602.py', 'output.jpg', 'c930d080-2407-11e7-807b-bd9de91b1602.py', '2e8ace70-2428-11 e7-807b-bd9de91b1602.py', '26492-1274250701.png', 'mytest.txt', '07fd1540-2411-11e7-807b-bd9de91b1602.py']
Explanation:
The ‘OS’ module provides a portable way of using operating system dependent functionality.
os.listdir(path='.'): Return a list containing the names of the entries in the directory given by path. The list is in arbitrary order, and does not include the special entries '.' and '..' even if they are present in the directory.
os.path.isfile(path): Return True if path is an existing regular file. This follows symbolic links, so both islink() and isfile() can be true for the same path.
os.path.join(path, *paths): Join one or more path segments intelligently. The return value is the concatenation of path and all members of *paths, with exactly one directory separator following each non-empty part, except the last.
The above Python code imports the ‘listdir’ and ‘isfile’ functions from the os module, and the ‘join’ function from the os.path module. It then uses the above three functions to generate a list of all the files in the directory /home/students. Finally print() function prints the resulting list of files.
Flowchart:
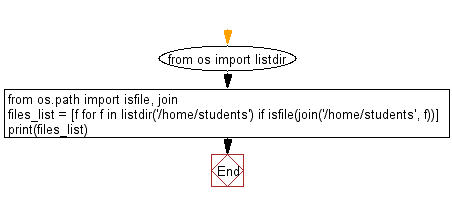
Python Code Editor:
Previous: Write a Python program to parse a string to Float or Integer.
Next: Write a Python program to print without newline or space?
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics