Python: Measure execution time
Python Basic: Exercise-57 with Solution
Write a Python program to get the execution time of a Python method.
Sample Solution:
Python Code:
# Import the 'time' module to work with time-related functions.
import time
# Define a function named 'sum_of_n_numbers' that calculates the sum of the first 'n' natural numbers.
def sum_of_n_numbers(n):
# Record the current time before the calculation.
start_time = time.time()
# Initialize a variable 's' to store the sum.
s = 0
# Loop through numbers from 1 to 'n' and accumulate the sum.
for i in range(1, n + 1):
s = s + i
# Record the current time after the calculation.
end_time = time.time()
# Return both the calculated sum and the time taken for the calculation.
return s, end_time - start_time
# Define the value of 'n' as 5.
n = 5
# Print the result, including the time taken to calculate the sum.
print("\nTime to sum of 1 to", n, "and required time to calculate is:", sum_of_n_numbers(n))
Sample Output:
Time to sum of 1 to 5 and required time to calculate is : (15, 2.384185791015625e-06)
Explanation:
The above Python code defines a function sum_of_n_numbers() that calculates the sum of the first n positive integers, and returns the sum and the time it took to calculate the sum.
time module - This module provides various time-related functions.
The sum_of_n_numbers() function uses a for loop to add up the first n positive integers and calculates the time it takes to do so using the time.time() function to get the start and end times of the operation. The function returns a tuple containing the sum of the first n positive integers and the time taken to calculate the sum.
Finally the code then calls the sum_of_n_numbers() function with an argument of n=5, and prints the result to the console using the print() function. The output displays the time it took to calculate the sum of the first 5 positive integers and the calculated sum.
Flowchart:
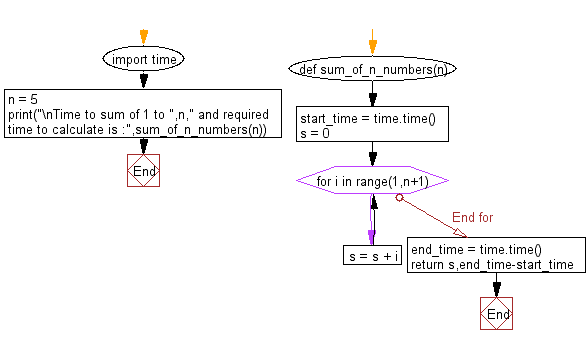
Python Code Editor:
Previous: Write a Python program to get height and the width of console window.
Next: Write a Python program to sum of the first n positive integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/python-basic-exercise-57.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics