Python: Convert pressure in kilopascals to pounds
Pressure Unit Converter
Write a Python program to convert pressure in kilopascals to pounds per square inch,a millimeter of mercury (mmHg) and atmosphere pressure.
Pictorial Presentation:
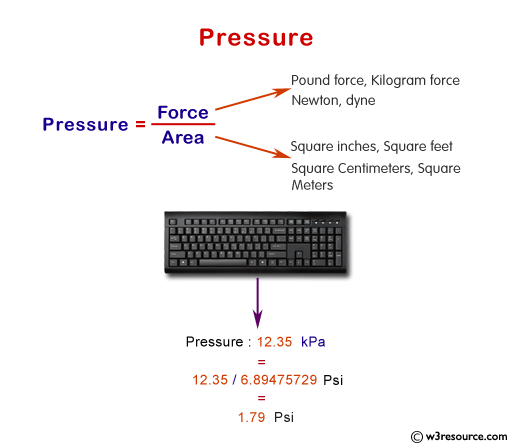
Sample Solution-1:
Python Code:
# Prompt the user to input pressure in kilopascals and convert it to a floating-point number.
kpa = float(input("Input pressure in kilopascals: "))
# Convert kilopascals to pounds per square inch (psi) using the conversion factor.
psi = kpa / 6.89475729
# Convert kilopascals to millimeters of mercury (mmHg) using the conversion factor.
mmhg = kpa * 760 / 101.325
# Convert kilopascals to atmospheres (atm) using the conversion factor.
atm = kpa / 101.325
# Print the pressure in pounds per square inch (psi) with 2 decimal places.
print("The pressure in pounds per square inch: %.2f psi" % (psi))
# Print the pressure in millimeters of mercury (mmHg) with 2 decimal places.
print("The pressure in millimeters of mercury: %.2f mmHg" % (mmhg))
# Print the atmosphere pressure (atm) with 2 decimal places.
print("Atmosphere pressure: %.2f atm." % (atm))
Sample Output:
Input pressure in in kilopascals> 12.35 The pressure in pounds per square inch: 1.79 psi The pressure in millimeter of mercury: 92.63 mmHg Atmosphere pressure: 0.12 atm.
Sample Solution-2:
Python Code:
# Prompt the user to input the pressure in kilopascals and store it as a floating-point number.
kpa = float(input("Input the pressure in kilopascals > "))
# Calculate the pressure in pounds per square inch (psi) and round it to 3 decimal places.
pressure_in_psi = round(kpa * 0.145038, 3)
# Calculate the pressure in millimeters of mercury (mmHg) and round it to 3 decimal places.
pressure_in_mmHg = round(kpa * 7.50062, 3)
# Calculate the pressure in standard atmospheres (atmp) and round it to 3 decimal places.
pressure_in_atmp = round(kpa * 0.0098692382432766, 3)
# Display the calculated pressure values in psi, mmHg, and atmp using an f-string.
print(f"Pressure = {pressure_in_psi} psi \nor {pressure_in_mmHg} mmHg \nor {pressure_in_atmp} atmp")
Sample Output:
Input the pressure in kilopascals > 12.35 Pressure = 1.791 psi or 92.633 mmHg or 0.122 atmp
For more Practice: Solve these Related Problems:
- Write a Python program to convert atmospheric pressure (atm) to Pascal, Torr, and bar.
- Write a Python program to convert pressure from bar to psi, mmHg, and kPa.
- Write a Python program to determine the air pressure at a given altitude.
- Write a Python program to calculate the difference in pressure between two given altitudes.
Go to:
Previous: Write a Python program to calculate body mass index.
Next: Write a Python program to calculate the sum of the digits in an integer.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.