Python: Concatenate N strings
Python Basic: Exercise-81 with Solution
Concatenate Strings
Write a Python program to concatenate N strings.
Pictorial Presentation:
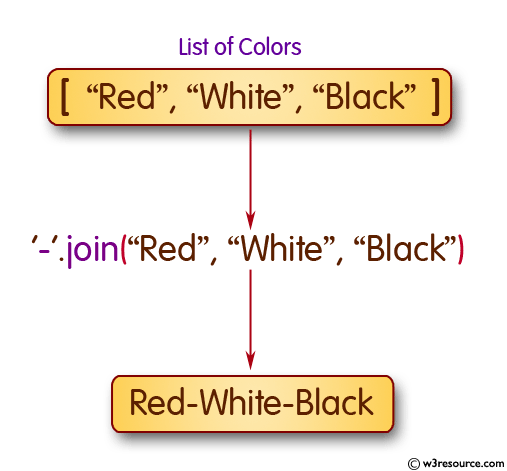
Sample Solution-1:
Python Code:
list_of_colors = ['Red', 'White', 'Black'] # Create a list of colors containing three elements
colors = '-'.join(list_of_colors) # Join the list elements with '-' and store the result in the 'colors' variable
print() # Print a blank line for spacing
print("All Colors: " + colors) # Print the concatenated colors with '-' between them
print() # Print another blank line for spacing
Sample Output:
All Colors: Red-White-Black
Sample Solution-2:
Concatenating With the + Operator:
Python Code:
# Print a description of the method being demonstrated
print("Concatenating With the + Operator:")
# Create a list of colors containing three elements
list_of_colors = ['Red', 'White', 'Black']
# Concatenate the list elements using the + operator and store the result in the 'colors' variable
colors = list_of_colors[0] + '-' + list_of_colors[1] + '-' + list_of_colors[2]
# Print a message and the concatenated 'colors' with '-' between them
print("All Colors: " + colors)
Sample Output:
Concatenating With the + Operator: All Colors: Red-White-Black
Python Code Editor :
Previous: Write a Python program to get the current value of the recursion limit.
Next: Write a Python program to calculate the sum of all items of a container (tuple, list, set, dictionary).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/python-basic-exercise-81.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics