Python Exercise: Convert temperatures to and from celsius, fahrenheit
2. Temperature Converter
Write a Python program to convert temperatures to and from Celsius and Fahrenheit.
Python: Centigrade and Fahrenheit Temperatures :
The centigrade scale, which is also called the Celsius scale, was developed by Swedish astronomer Andres Celsius. In the centigrade scale, water freezes at 0 degrees and boils at 100 degrees. The centigrade to Fahrenheit conversion formula is:
Fahrenheit and centigrade are two temperature scales in use today. The Fahrenheit scale was developed by the German physicist Daniel Gabriel Fahrenheit . In the Fahrenheit scale, water freezes at 32 degrees and boils at 212 degrees.
C = (5/9) * (F - 32)
where F is the Fahrenheit temperature. You can also use this Web page to convert Fahrenheit temperatures to centigrade. Just enter a Fahrenheit temperature in the text box below, then click on the Convert button.
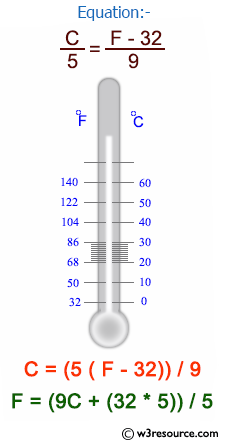
Sample Solution:
Python Code:
# Prompt the user to input a temperature in the format (e.g., 45F, 102C, etc.)
temp = input("Input the temperature you like to convert? (e.g., 45F, 102C etc.) : ")
# Extract the numerical part of the temperature and convert it to an integer
degree = int(temp[:-1])
# Extract the convention part of the temperature input (either 'C' or 'F')
i_convention = temp[-1]
# Check if the input convention is in uppercase 'C' (Celsius)
if i_convention.upper() == "C":
# Convert the Celsius temperature to Fahrenheit
result = int(round((9 * degree) / 5 + 32))
o_convention = "Fahrenheit" # Set the output convention as Fahrenheit
# Check if the input convention is in uppercase 'F' (Fahrenheit)
elif i_convention.upper() == "F":
# Convert the Fahrenheit temperature to Celsius
result = int(round((degree - 32) * 5 / 9))
o_convention = "Celsius" # Set the output convention as Celsius
else:
# If the input convention is neither 'C' nor 'F', print an error message and exit the program
print("Input proper convention.")
quit()
# Display the converted temperature in the specified output convention
print("The temperature in", o_convention, "is", result, "degrees.")
Sample Output:
Input the temperature you like to convert? (e.g., 45F, 102C etc.) : 104f The temperature in Celsius is 40 degrees.
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Python program to convert a list of temperatures in Celsius to Fahrenheit and vice versa, outputting both results side-by-side.
- Write a Python program that accepts a temperature string (e.g., "60C" or "45F") and converts it to the opposite scale.
- Write a Python program to implement temperature conversion using lambda functions and map() for a list of mixed temperature values.
- Write a Python program to validate user input for temperature conversion and re-prompt if the input format is invalid.
Go to:
Previous: Write a Python program to find those numbers which are divisible by 7 and multiple of 5, between 1500 and 2700 (both included).
Next: Write a Python program to guess a number between 1 to 9.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.