Python Exercise: Create the multiplication table of a number
43. Multiplication Table
Write a Python program to create the multiplication table (from 1 to 10) of a number.
Pictorial Presentation:
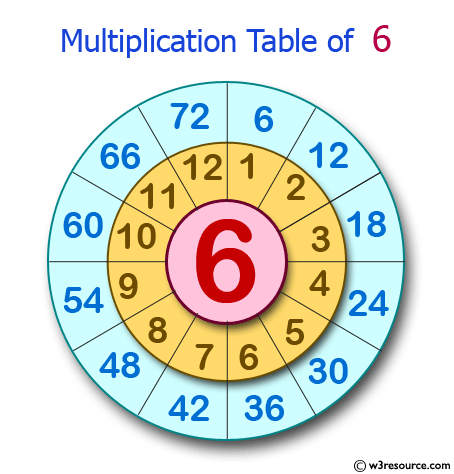
Sample Solution:
Python Code:
# Prompt the user to input a number and convert it to an integer, assigning it to the variable 'n'
n = int(input("Input a number: "))
# Use a for loop to iterate 10 times, starting from 1 up to 10 (excluding 11)
for i in range(1, 11):
# Print the multiplication table for the entered number 'n' by multiplying it with each iteration 'i'
print(n, 'x', i, '=', n * i)
Sample Output:
Input a number: 5 5 x 1 = 5 5 x 2 = 10 5 x 3 = 15 5 x 4 = 20 5 x 5 = 25 5 x 6 = 30 5 x 7 = 35 5 x 8 = 40 5 x 9 = 45 5 x 10 = 50
Flowchart :
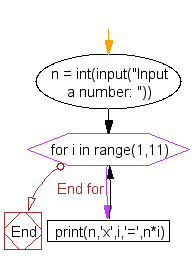
For more Practice: Solve these Related Problems:
- Write a Python program to generate the multiplication table of a given number using a for loop.
- Write a Python program to format and print the multiplication table for an input number from 1 to 10.
- Write a Python program to use nested loops to create and display the multiplication table for a single number.
- Write a Python program to create a function that prints the multiplication table of a number, with proper alignment of columns.
Go to:
Previous: Write a Python program to calculate the sum and average of n integer numbers (input from the user). Input 0 to finish.
Next: Write a Python program to construct the following pattern, using a nested loop number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.