Python Exercise: Multiply all the numbers in a list
3. Multiply All Numbers in a List
Write a Python function to multiply all the numbers in a list.
Sample Solution:
Python Code:
# Define a function named 'multiply' that takes a list of numbers as input
def multiply(numbers):
# Initialize a variable 'total' to store the multiplication result, starting at 1
total = 1
# Iterate through each element 'x' in the 'numbers' list
for x in numbers:
# Multiply the current element 'x' with the 'total'
total *= x
# Return the final multiplication result stored in the 'total' variable
return total
# Print the result of calling the 'multiply' function with a tuple of numbers (8, 2, 3, -1, 7)
print(multiply((8, 2, 3, -1, 7)))
Sample Output:
-336
Explanation:
In the exercise above the code defines a function named "multiply()" that takes a list of numbers as input and returns the product of all the numbers in the list. The final print statement demonstrates the result of calling the "multiply()" function with a tuple containing the numbers (8, 2, 3, -1, 7).
Pictorial presentation:
Flowchart:
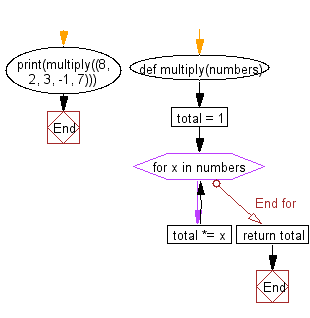
For more Practice: Solve these Related Problems:
- Write a Python function that iterates over a list with a for-loop and multiplies all the numbers together.
- Write a Python function that uses recursion to calculate the product of all numbers in a list.
- Write a Python function that uses functools.reduce() to multiply all elements in a list and return the result.
- Write a Python function that multiplies all numbers in a list but skips zeros to avoid nullifying the product.
Go to:
Previous: Write a Python function to sum all the numbers in a list.
Next: Write a Python program to reverse a string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.