Python Exercise: Check whether a number falls in a given range
6. Check if a Number Falls Within a Given Range
Write a Python function to check whether a number falls within a given range.
Sample Solution-1:
Python Code:
# Define a function named 'test_range' that checks if a number 'n' is within the range 3 to 8 (inclusive)
def test_range(n):
# Check if 'n' is within the range from 3 to 8 (inclusive) using the 'in range()' statement
if n in range(3, 9):
# If 'n' is within the range, print that 'n' is within the given range
print("%s is in the range" % str(n))
else:
# If 'n' is outside the range, print that the number is outside the given range
print("The number is outside the given range.")
# Call the 'test_range' function with the argument 5
test_range(5)
Sample Output:
5 is in the range
Explanation:
In the exercise above the code defines a function called "test_range()" that checks if the given number 'n' falls within the specified range (from 3 to 8). It then calls this function with the argument 5 and prints whether the number is within or outside the specified range.
Pictorial presentation:
Flowchart:
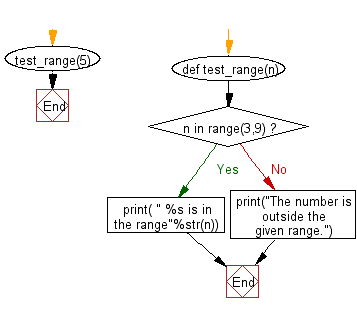
Sample Solution-2:
- Use arithmetic comparison to check if the given number is in the specified range.
- If the second parameter, end, is not specified, the range is considered to be from 0 to start.
Python Code:
# Define a function named 'test_range' that checks if a number 'n' is within a specified range.
# The range is determined by 'start_num' and 'end_num' (with 'end_num' having a default value of 0)
def test_range(n, start_num, end_num=0):
# Check if 'end_num' is greater than or equal to 'start_num'
# If true, use 'start_num <= n <= end_num' to determine if 'n' is within the range
# If false, swap 'end_num' and 'start_num' and check 'end_num <= n <= start_num'
return start_num <= n <= end_num if end_num >= start_num else end_num <= n <= start_num
# Print the result of checking if 5 is within the range from 2 to 7 (inclusive)
print(test_range(5, 2, 7))
# Print the result of checking if 5 is within the default range from 7 to 0 (inclusive)
print(test_range(5, 7))
# Print the result of checking if 1 is within the range from 3 to 6 (inclusive)
print(test_range(1, 3, 6))
# Print the result of checking if 6 is within the range from 5 to the default value 0 (inclusive)
print(test_range(6, 5))
Sample Output:
True True False False
Explanation:
In the exercise above the code defines a function "test_range()" that checks if a given number n falls within a specified range (start_num to end_num). If end_num is not provided, it defaults to 0. The function then performs a range check and prints the results of various range checks involving different combinations of input values.
Flowchart:
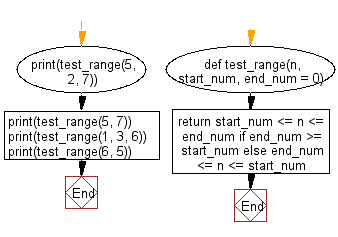
For more Practice: Solve these Related Problems:
- Write a Python function that accepts a number and two boundaries, and returns True if the number is between them (inclusive), otherwise False.
- Write a Python function that uses a ternary operator to check if a number is within a specified range and returns a custom message.
- Write a Python function that validates input types and then checks if the number falls within the range, raising an exception if not.
- Write a Python function that uses lambda to create a quick range-checking function and tests it on various inputs.
Go to:
Previous: Write a Python function to calculate the factorial of a number (a non-negative integer). The function accepts the number as an argument.
Next: Write a Python function that accepts a string and calculate the number of upper case letters and lower case letters.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.