Python Exercise: Unique elements from a list
Python Functions: Exercise-8 with Solution
Write a Python function that takes a list and returns a new list with distinct elements from the first list.
Sample Solution:
Python Code:
# Define a function named 'unique_list' that takes a list 'l' as input and returns a list of unique elements
def unique_list(l):
# Create an empty list 'x' to store unique elements
x = []
# Iterate through each element 'a' in the input list 'l'
for a in l:
# Check if the element 'a' is not already present in the list 'x'
if a not in x:
# If 'a' is not in 'x', add it to the list 'x'
x.append(a)
# Return the list 'x' containing unique elements
return x
# Print the result of calling the 'unique_list' function with a list containing duplicate elements
print(unique_list([1, 2, 3, 3, 3, 3, 4, 5]))
Sample Output:
[1, 2, 3, 4, 5]
Explanation:
In the exercise above the code defines a function named "unique_list()" that takes a list as input and returns a new list containing only the unique elements from the input list. It uses a loop to iterate through the input list and checks whether each element is already present in the new list 'x'. If an element is not found in 'x', it appends it to 'x'. Finally, it demonstrates the function by calling it with a list of duplicate elements [1, 2, 3, 3, 3, 3, 4, 5] and prints the resulting list with unique elements.
Pictorial presentation:
Flowchart:
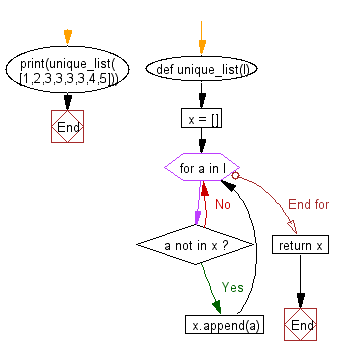
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python function that accepts a string and calculate the number of upper case letters and lower case letters.
Next: Write a Python function that takes a number as a parameter and check the number is prime or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/python-functions-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics