Python: Check that a string contains only a certain set of characters
1. Allowed Characters Check
Write a Python program to check that a string contains only a certain set of characters (in this case a-z, A-Z and 0-9).
Sample Solution:
Python Code:
import re
def is_allowed_specific_char(string):
charRe = re.compile(r'[^a-zA-Z0-9]')
string = charRe.search(string)
return not bool(string)
print(is_allowed_specific_char("ABCDEFabcdef123450"))
print(is_allowed_specific_char("*&%@#!}{"))
Sample Output:
True False
Pictorial Presentation:
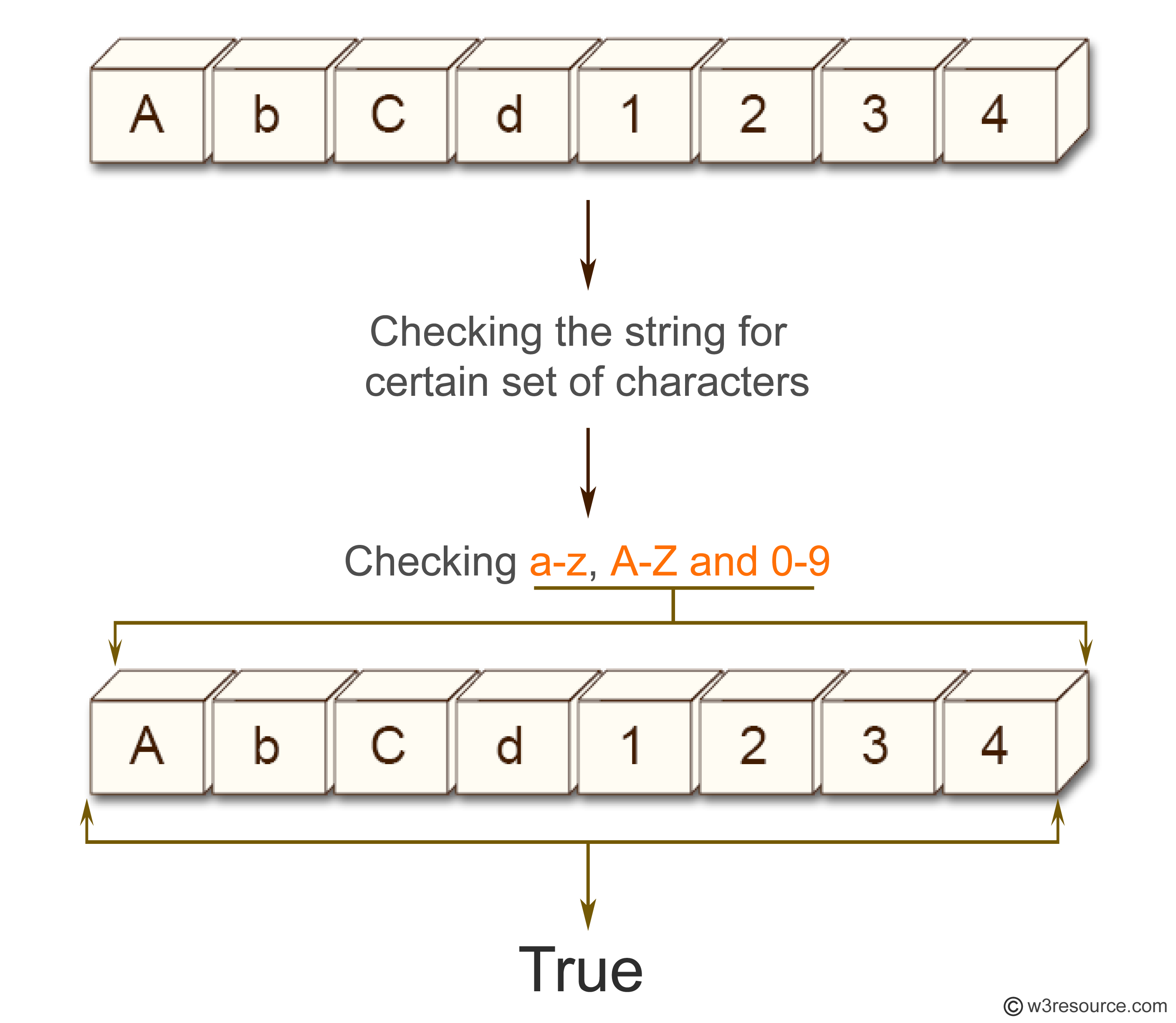
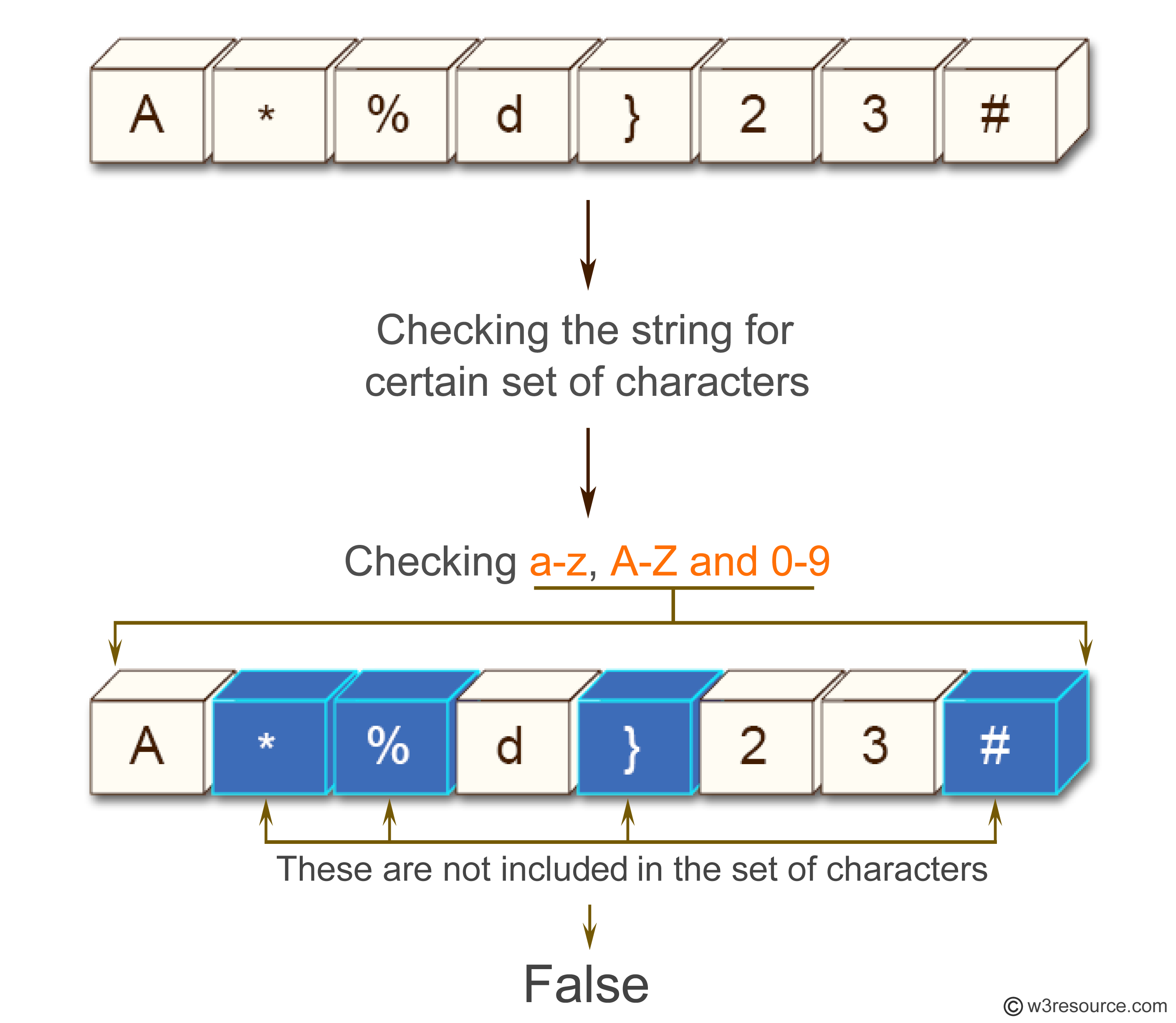
Flowchart:
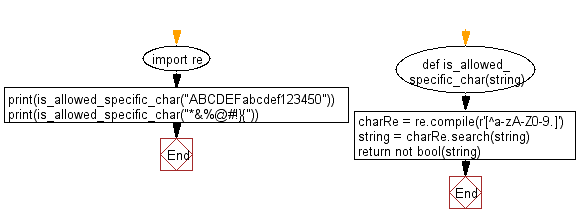
For more Practice: Solve these Related Problems:
- Write a Python program to validate that a string contains only lowercase letters and digits.
- Write a Python program to check if a string consists solely of alphanumeric characters and underscores.
- Write a Python program to verify that a string contains only the characters a–z, A–Z, 0–9, and no special symbols.
- Write a Python program to determine if a string matches a regex pattern that allows only letters and numbers, then count the frequency of each character.
Go to:
Previous: Python Regular Expression Home.
Next: Write a Python program that matches a string that has an a followed by zero or more b's.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.