Python: Matches a string that has an a followed by three 'b'
5. a Followed by Exactly 3 b's
Write a Python program that matches a string that has an a followed by three 'b'.
Sample Solution:
Python Code:
import re
def text_match(text):
patterns = 'ab{3}?'
if re.search(patterns, text):
return 'Found a match!'
else:
return('Not matched!')
print(text_match("abbb"))
print(text_match("aabbbbbc"))
Sample Output:
Found a match! Found a match!
Pictorial Presentation:
Flowchart:
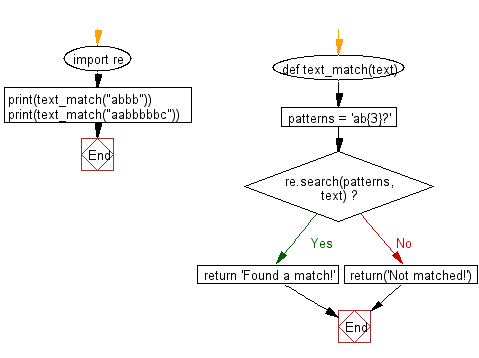
For more Practice: Solve these Related Problems:
- Write a Python program to match strings that contain an 'a' immediately followed by exactly three 'b's.
- Write a Python script to filter a list of strings to return only those that have the pattern 'abbb'.
- Write a Python program to check whether a string exactly matches the pattern 'abbb' and print the result.
- Write a Python program to extract and print all substrings that match an 'a' followed by exactly three 'b's from a given text.
Go to:
Previous: Write a Python program that matches a string that has an a followed by zero or one 'b'.
Next: Write a Python program that matches a string that has an a followed by two to three 'b'.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.