Python: Remove lowercase substrings from a given string
53. Remove Lowercase Substrings
Write a Python program to remove lowercase substrings from a given string.
Sample Solution:
Python Code:
import re
str1 = 'KDeoALOklOOHserfLoAJSIskdsf'
print("Original string:")
print(str1)
print("After removing lowercase letters, above string becomes:")
remove_lower = lambda text: re.sub('[a-z]', '', text)
result = remove_lower(str1)
print(result)
Sample Output:
Original string: KDeoALOklOOHserfLoAJSIskdsf After removing lowercase letters, above string becomes: KDALOOOHLAJSI
Flowchart:
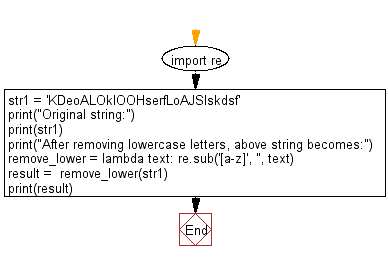
For more Practice: Solve these Related Problems:
- Write a Python program to remove all contiguous sequences of lowercase letters from a string and print the result.
- Write a Python script to delete any substring composed entirely of lowercase letters from a given text.
- Write a Python program to search for and eliminate lowercase-only words from a sentence using regex.
- Write a Python program to strip out lowercase substrings from a mixed-case string and output the modified string.
Go to:
Previous: Write a Python program to insert spaces between words starting with capital letters.
Next: Write a Python program to concatenate the consecutive numbers in a given string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.