Python: Take any number of iterable objects or objects with a length property and returns the longest one
56. Longest Iterable
Write a Python program that takes any number of iterable objects or objects with a length property and returns the longest one.
- Use max() with len() as the key to return the item with the greatest length.
- If multiple objects have the same length, the first one will be returned.
Sample Solution:
Python Code:
def longest_item(*args):
return max(args, key = len)
print(longest_item('Red', 'Green', 'Black', 'Orange'))
print(longest_item([1, 2, 3], [1, 2, 3, 4], [1, 2, 3, 4, 5]))
print(longest_item([1, 2, 3], 'Java'))
print(longest_item({10, 100}, 'Python'))
Sample Output:
Orange [1, 2, 3, 4, 5] Java Python
Flowchart:
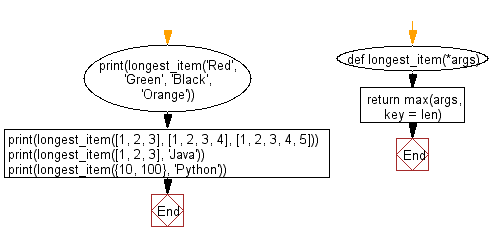
For more Practice: Solve these Related Problems:
- Write a Python program that accepts multiple iterables and returns the one with the greatest length.
- Write a Python script to compare several sequences and output the longest one among them.
- Write a Python program to take any number of iterables and then determine which has the maximum number of elements.
- Write a Python function to iterate over a collection of sequences and return the sequence with the highest length property.
Go to:
Previous Python Exercise: Convert a given string to snake case.
Next Python Exercise: Match beginning and end of a word with a vowel.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.