Python: Check if a set is a subset of another set
10. Check if a Set is a Subset of Another Set
Write a Python program to check if a set is a subset of another set.
From Wikipedia,
In mathematics, a set A is a subset of a set B if all elements of A are also elements of B; B is then a superset of A. It is possible for A and B to be equal; if they are unequal, then A is a proper subset of B. The relationship of one set being a subset of another is called inclusion (or sometimes containment). A is a subset of B may also be expressed as B includes (or contains) A or A is included (or contained) in B.
Visual Presentation:
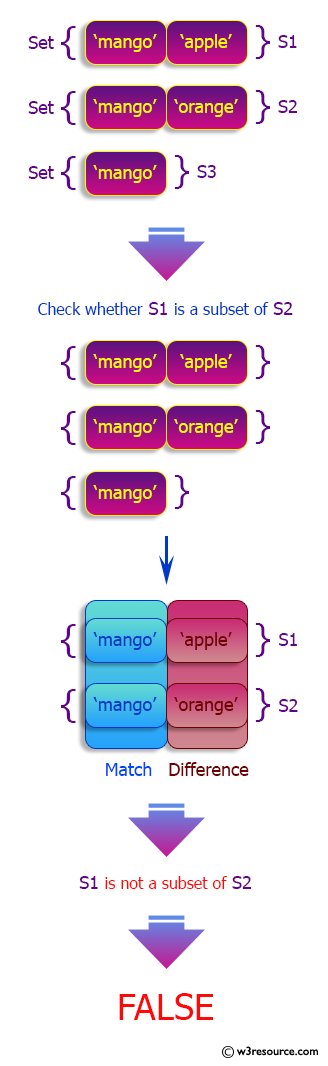
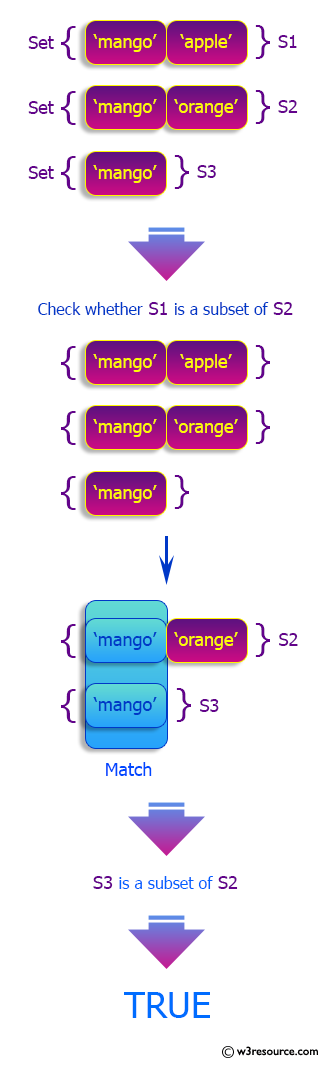
Sample Solution:
Python Code:
# Print a message to describe the purpose of the code.
print("Check if a set is a subset of another set, using comparison operators and issubset():\n")
# Create sets 'setx', 'sety', and 'setz' with different elements.
setx = set(["apple", "mango"])
sety = set(["mango", "orange"])
setz = set(["mango"])
# Print the contents of sets 'setx', 'sety', and 'setz'.
print("x: ", setx)
print("y: ", sety)
print("z: ", setz, "\n")
# Check if 'setx' is a subset of 'sety' and print the result using both comparison operators and 'issubset()'.
print("If x is a subset of y")
print(setx <= sety)
print(setx.issubset(sety))
# Check if 'sety' is a subset of 'setx' and print the result using both comparison operators and 'issubset()'.
print("If y is a subset of x")
print(sety <= setx)
print(sety.issubset(setx))
# Check if 'sety' is a subset of 'setz' and print the result using both comparison operators and 'issubset()'.
print("\nIf y is a subset of z")
print(sety <= setz)
print(sety.issubset(setz))
# Check if 'setz' is a subset of 'sety' and print the result using both comparison operators and 'issubset()'.
print("If z is a subset of y")
print(setz <= sety)
print(setz.issubset(sety))
Sample Output:
Check if a set is a subset of another set, using comparison operators and issubset(): x: {'mango', 'apple'} y: {'mango', 'orange'} z: {'mango'} If x is subset of y False False If y is subset of x False False If y is subset of z False False If z is subset of y True True
For more Practice: Solve these Related Problems:
- Write a Python program to check if one set is a subset of another using the issubset() method.
- Write a Python program to compare two sets using the <= operator to determine subset relation.
- Write a Python program to implement a function that returns True if a given set is entirely contained in another set.
- Write a Python program to use set intersection and comparison to verify the subset relationship between two sets.
Go to:
Previous: Write a Python program to create a symmetric difference.
Next: Write a Python program to create a shallow copy of sets.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.