Python: Add 'ing' at the end of a given string (length should be at least 3). If the given string already ends with 'ing' then add 'ly' instead. If the string length of the given string is less than 3, leave it unchanged
Python String: Exercise-6 with Solution
Add ing or ly to a string.
Write a Python program to add 'ing' at the end of a given string (length should be at least 3). If the given string already ends with 'ing' then add 'ly' instead. If the string length of the given string is less than 3, leave it unchanged.
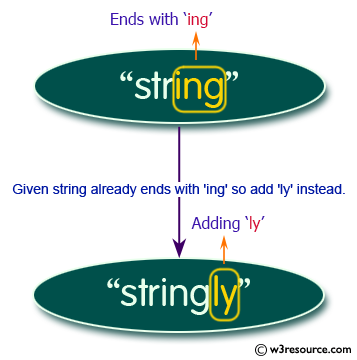
Sample Solution:
Python Code:
# Define a function named add_string that takes one argument, 'str1'.
def add_string(str1):
# Get the length of the input string 'str1' and store it in the variable 'length'.
length = len(str1)
# Check if the length of 'str1' is greater than 2 characters.
if length > 2:
# If the last three characters of 'str1' are 'ing', add 'ly' to the end.
if str1[-3:] == 'ing':
str1 += 'ly'
else:
# If the last three characters are not 'ing', add 'ing' to the end.
str1 += 'ing'
# Return the modified 'str1'.
return str1
# Call the add_string function with different input strings and print the results.
print(add_string('ab')) # Output: 'ab'
print(add_string('abc')) # Output: 'abcing'
print(add_string('string')) # Output: 'stringly'
Sample Output:
ab abcing stringly
Flowchart:
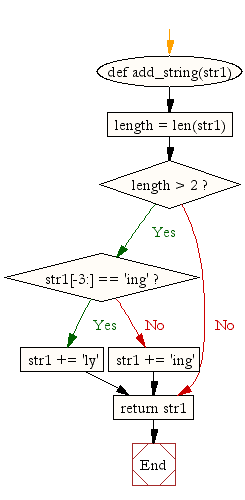
Python Code Editor:
Previous: Write a Python program to get a single string from two given strings, separated by a space and swap the first two characters of each string.
Next: Write a Python program to find the first appearance of the substring 'not' and 'poor' from a given string, if 'bad' follows the 'poor', replace the whole 'not'...'poor' substring with 'good'. Return the resulting string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/string/python-data-type-string-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics