Customizing Tkinter radio buttons with distinct styles
Write a Python program that creates a set of custom radio buttons with distinct styles for selection and deselection using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
class TraditionalRadioButtons:
def __init__(self, root):
self.root = root
self.root.title("Traditional Radio Buttons")
# Initialize the selected radio button index
self.selected_option = tk.IntVar()
self.selected_option.set(0) # Initialize as no option selected
# Create traditional radio buttons
self.radio_button1 = tk.Radiobutton(root, text="Option 1", variable=self.selected_option, value=1, command=self.display_message)
self.radio_button2 = tk.Radiobutton(root, text="Option 2", variable=self.selected_option, value=2, command=self.display_message)
self.radio_button3 = tk.Radiobutton(root, text="Option 3", variable=self.selected_option, value=3, command=self.display_message)
# Pack the radio buttons
self.radio_button1.pack(pady=10)
self.radio_button2.pack(pady=10)
self.radio_button3.pack(pady=10)
def display_message(self):
selected_value = self.selected_option.get()
if selected_value == 1:
message = "Option 1 selected!"
elif selected_value == 2:
message = "Option 2 selected!"
elif selected_value == 3:
message = "Option 3 selected!"
else:
message = "No option selected!"
messagebox.showinfo("Radio Button Selection", message)
if __name__ == "__main__":
root = tk.Tk()
app = TraditionalRadioButtons(root)
root.mainloop()
Explanation:
In the exercise above -
- It imports the required modules, "tkinter" and "messagebox".
- The "TraditionalRadioButtons" class is defined to create and manage GUI elements.
- Inside the class constructor (init), it sets up the main window and initializes a variable called 'selected_option' to store the selected radio button's value.
- Three radio buttons (radio_button1, radio_button2, and radio_button3) are created using the "Radiobutton" widget. A radio button has a specific value (1, 2, or 3) and a callback function (display_message) for handling selections.
- The radio buttons are packed (arranged vertically) in the main window.
- The "display_message()" function checks the selected radio button's value and displays a corresponding message in a message box (messagebox.showinfo).
- The code creates an instance of the "TraditionalRadioButtons" class and starts the Tkinter main loop to run the application.
Output:
Flowchart:
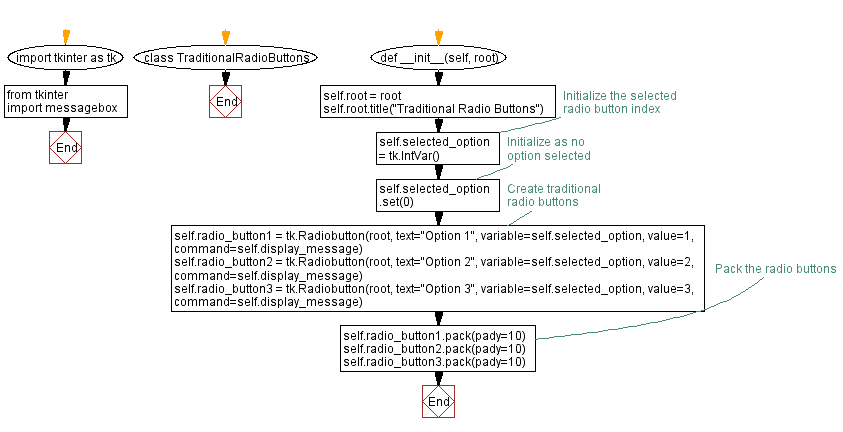
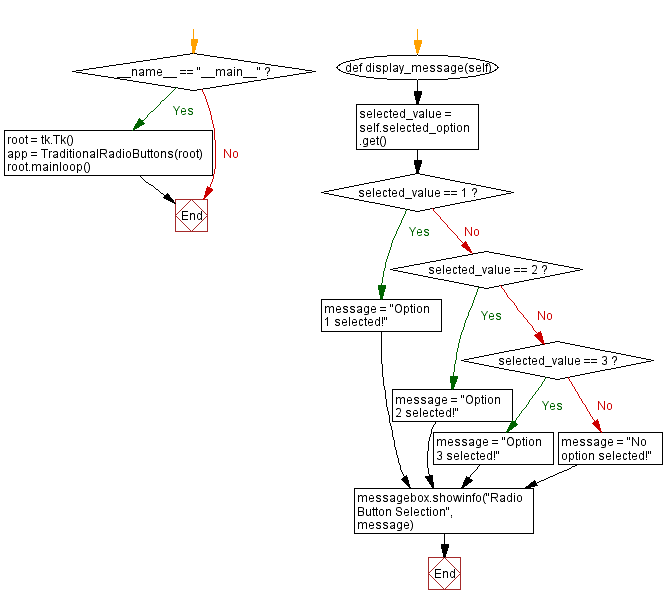
Go to:
Previous: Customizing Tkinter entry Widgets with themes.
Next: Customizing Tkinter slider widget with a unique theme.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.