Python tkinter widgets Exercise: Create two buttons exit and hello using tkinter module
Write a Python GUI program to create two buttons exit and hello using tkinter module.
On pressing hello button print the text “Tkinter is easy to create GUI” on the terminal.
Sample Solution:
Python Code:
import tkinter as tk
def write_text():
print("Tkinter is easy to create GUI!")
parent = tk.Tk()
frame = tk.Frame(parent)
frame.pack()
text_disp= tk.Button(frame,
text="Hello",
command=write_text
)
text_disp.pack(side=tk.LEFT)
exit_button = tk.Button(frame,
text="Exit",
fg="green",
command=quit)
exit_button.pack(side=tk.RIGHT)
parent.mainloop()
Explanation:
In the exercise above -
- import tkinter as tk - Import the Tkinter library (tkinter).
- def write_text(): - Define a function (write_text) to print a message.
- parent = tk.Tk() - Create the main Tkinter window (parent).
- frame = tk.Frame(parent) - Create a Frame widget (frame) within the main window.
- text_disp= tk.Button(frame, text="Hello", command=write_text) - Create a "Hello" button (text_disp) that calls the write_text function when clicked.
- exit_button = tk.Button(frame, text="Exit", fg="green", command=quit) - Create an "Exit" button (exit_button) to close the application when clicked.
- exit_button.pack(side=tk.RIGHT) - Display the buttons within the Frame (frame).
- parent.mainloop() - Start the Tkinter main loop to run the GUI application.
Sample Output:
Flowchart:
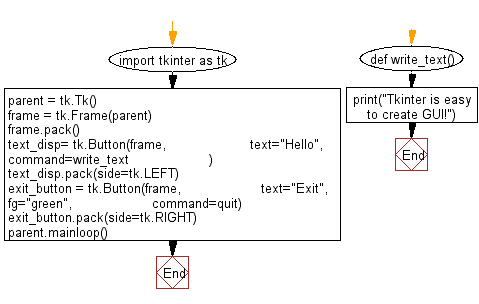
Go to:
Previous: Write a Python GUI program to add a canvas in your application using tkinter module.
Next: Write a Python GUI program to create a Combobox with three options using tkinter module.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.