Python Exercise: Find the index of an item of a tuple
Python tuple: Exercise-14 with Solution
Write a Python program to find the index of an item in a tuple.
Visual Presentation:
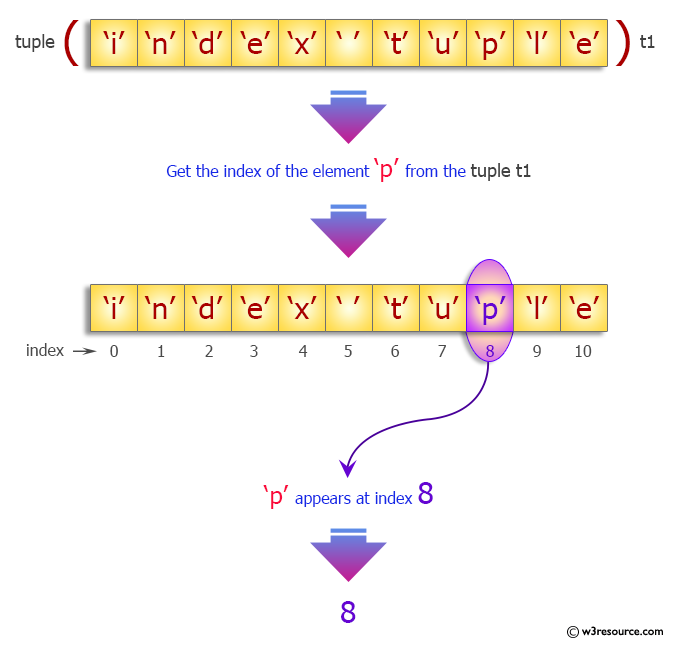
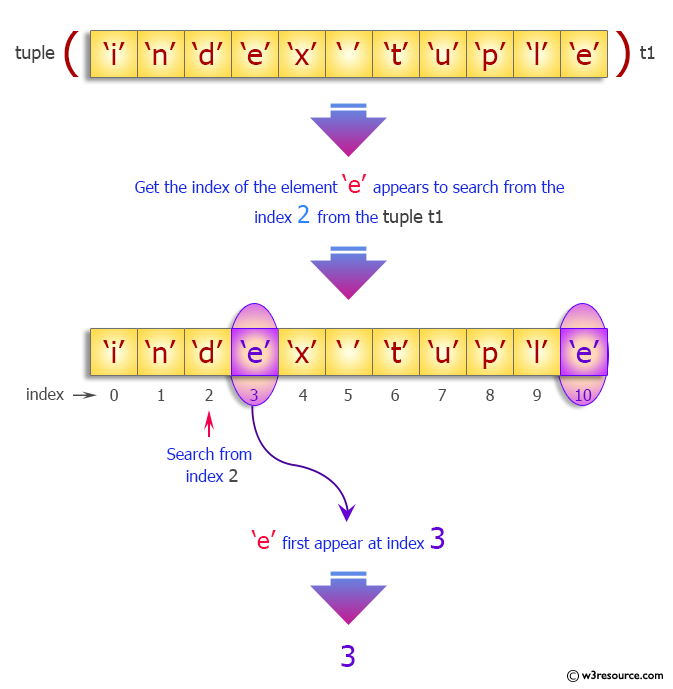
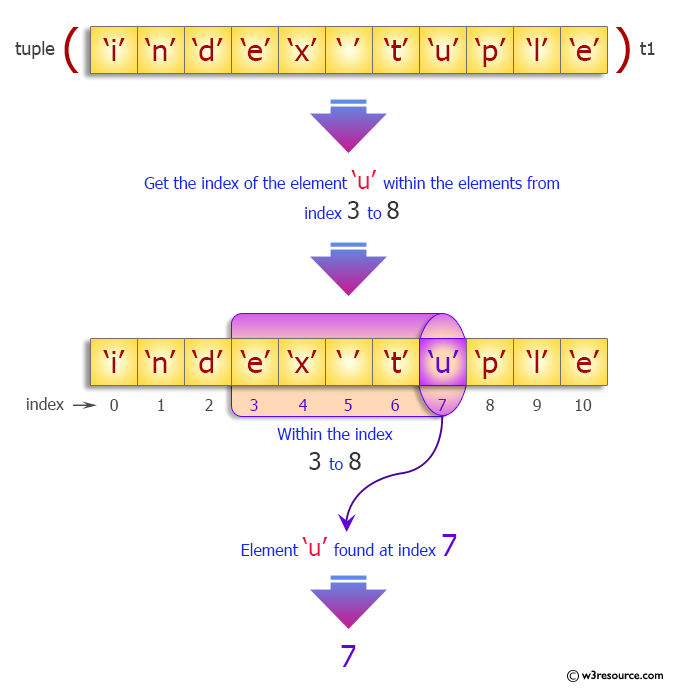
Sample Solution:
Python Code:
# Create a tuple by converting the string "index tuple" into a tuple
tuplex = tuple("index tuple")
# Print the contents of the 'tuplex' tuple
print(tuplex)
# Get the index of the first occurrence of the character "p" in the 'tuplex' tuple
index = tuplex.index("p")
# Print the index value
print(index)
# Define the index from which you want to start searching for the character "p" (index 5)
index = tuplex.index("p", 5)
# Print the index value
print(index)
# Define a segment of the 'tuplex' tuple (from index 3 to 6) within which you want to search for the character "e"
index = tuplex.index("e", 3, 6)
# Print the index value
print(index)
# Attempt to find the index of the character "y," which does not exist in the 'tuplex' tuple
# This will raise a "ValueError" exception because the item is not in the tuple
index = tuplex.index("y")
Sample Output:
('i', 'n', 'd', 'e', 'x', ' ', 't', 'u', 'p', 'l', 'e') 8 8 3 Traceback (most recent call last): File "d0e5ee40-30ab-11e7-a6a0-0b37d4d0b2c6.py", line 14, in <module> index = tuplex.index("y") ValueError: tuple.index(x): x not in tuple
Flowchart:
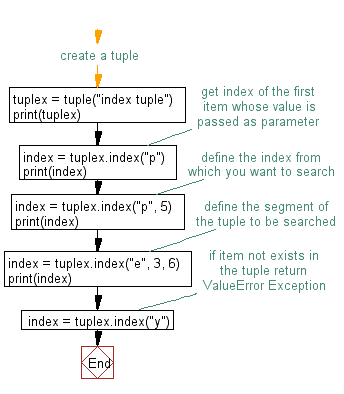
Python Code Editor:
Previous: Write a Python program to slice a tuple.
Next: Write a Python program to find the length of a tuple.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/tuple/python-tuple-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics