Python Exercise: Check if a specified element presents in a tuple of tuples
30. Check if a Specified Element Appears in a Tuple of Tuples
Write a Python program to check if a specified element appears in a tuple of tuples.
Sample Solution:
Python Code:
# Define a function named 'check_in_tuples' that takes a tuple of tuples 'colors' and a string 'c' as input.
def check_in_tuples(colors, c):
# Use the 'any' function to check if the string 'c' is present in any of the inner tuples within 'colors'.
result = any(c in tu for tu in colors)
# Return the result, which is a Boolean indicating whether 'c' is found in any of the inner tuples.
return result
# Create a tuple of tuples 'colors', where each inner tuple represents a group of colors.
colors = (
('Red', 'White', 'Blue'),
('Green', 'Pink', 'Purple'),
('Orange', 'Yellow', 'Lime'),
)
# Print a message indicating the original list of color tuples.
print("Original list:")
print(colors)
# Define a string 'c1' to check its presence in the color tuples.
c1 = 'White'
# Print a message indicating the check for the presence of 'c1' in the tuples of tuples.
print("\nCheck if", c1, "present in said tuple of tuples!")
# Call the 'check_in_tuples' function to check if 'c1' is present in any of the inner tuples and print the result.
print(check_in_tuples(colors, c1))
# Redefine 'c1' to check its presence again.
c1 = 'White'
# Print a message indicating the check for the presence of 'c1' in the tuples of tuples.
print("\nCheck if", c1, "present in said tuple of tuples!")
# Call the 'check_in_tuples' function to check if 'c1' is present in any of the inner tuples and print the result.
print(check_in_tuples(colors, c1))
# Redefine 'c1' to check its presence once more with a different value.
c1 = 'Olive'
# Print a message indicating the check for the presence of 'c1' in the tuples of tuples.
print("\nCheck if", c1, "present in said tuple of tuples!")
# Call the 'check_in_tuples' function to check if 'c1' is present in any of the inner tuples and print the result.
print(check_in_tuples(colors, c1))
Sample Output:
Original list: (('Red', 'White', 'Blue'), ('Green', 'Pink', 'Purple'), ('Orange', 'Yellow', 'Lime')) Check if White presenet in said tuple of tuples! True Check if White presenet in said tuple of tuples! True Check if Olive presenet in said tuple of tuples! False
Flowchart:
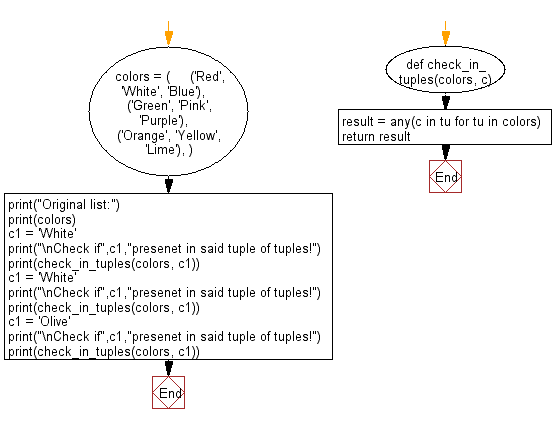
For more Practice: Solve these Related Problems:
- Write a Python program to search for a specific element in each tuple of a tuple of tuples and return True if found.
- Write a Python program to iterate over a tuple of tuples and determine if a target element exists in any of the inner tuples.
- Write a Python program to use nested loops to check for the presence of an element in a tuple of tuples and output a boolean result.
- Write a Python program to implement a function that returns the tuple index and inner index if a specified element is found.
Go to:
Previous: Write a Python program to convert a given tuple of positive integers into an integer.
Next: Write a Python program to compute element-wise sum of given tuples.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.