Python unit test: Check list sorting
2. Unit Test for Sorted List in Ascending Order
Write a Python unit test program to check if a list is sorted in ascending order.
Sample Solution:
Python Code:
# Import the 'unittest' module for writing unit tests.
import unittest
# Define a function 'is_sorted_ascending' to check if a list is sorted in ascending order.
def is_sorted_ascending(lst):
# Check if all elements at index i are less than or equal to elements at index i+1.
return all(lst[i] <= lst[i+1] for i in range(len(lst)-1)
# Define a test case class 'TestSortedAscending' that inherits from 'unittest.TestCase'.
class TestSortedAscending(unittest.TestCase):
# Define a test method 'test_sorted_list' to test a sorted list.
def test_sorted_list(self):
# Uncomment one of the 'lst' lines for testing a sorted or unsorted list.
#lst = [5, 7, 2, 8, 1, 9]
lst = [1, 2, 3, 4, 5, 6, 7]
print("Sorted list: ", lst)
# Assert that the list is sorted in ascending order.
self.assertTrue(is_sorted_ascending(lst), "The list is not sorted in ascending order")
# Define a test method 'test_unsorted_list' to test an unsorted list.
def test_unsorted_list(self):
# Uncomment one of the 'lst' lines for testing a sorted or unsorted list.
#lst = [1, 2, 3]
lst = [5, 7, 2, 8, 1, 9]
print("Unsorted list: ", lst)
# Assert that the list is not sorted in ascending order.
self.assertFalse(is_sorted_ascending(lst), "The list is sorted in ascending order")
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
Ran 2 tests in 0.001s FAILED (failures=2) Sorted list: [5, 7, 2, 8, 1, 9] Unsorted list: [1, 2, 3]
Ran 2 tests in 0.003s OK Sorted list: [1, 2, 3, 4, 5, 6, 7] Unsorted list: [5, 7, 2, 8, 1, 9]
Explanation:
In the above exercise, is_sorted_ascending(lst) function takes a list as input and returns True if the list is sorted in ascending order, and False otherwise. The TestSortedAscending class inherits from unittest.TestCase, and defines two test methods: test_sorted_list and test_unsorted_list.
Flowchart:
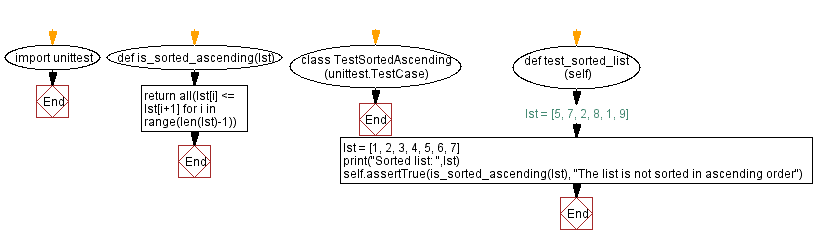
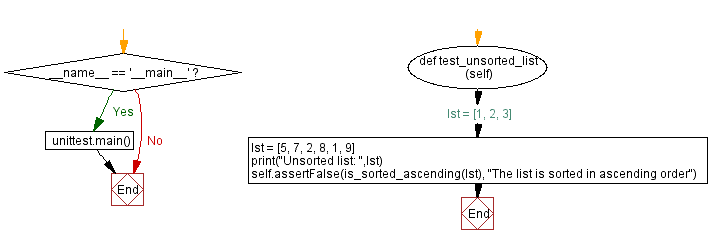
For more Practice: Solve these Related Problems:
- Write a Python unit test program that uses assertListEqual to verify a function correctly identifies a list sorted in ascending order.
- Write a Python unit test program that checks that an unsorted list is correctly detected as not being sorted in ascending order.
- Write a Python unit test program that tests the sorted order of lists with duplicate and single elements.
- Write a Python unit test program that confirms a sorting function leaves an already sorted list unchanged.
Go to:
Previous: Check prime number.
Next: Check equality of two lists.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.