Python unit test: Check file existence in a directory
Python Unit test: Exercise-5 with Solution
Write a Python unit test program to check if a file exists in a specified directory.
Sample Solution:
Python Code:
# Import the 'os' and 'unittest' modules for working with the file system and writing unit tests.
import os
import unittest
# Define a function 'file_exists' to check if a file exists in a specified directory.
def file_exists(directory, filename):
# Create the full file path by joining the directory and filename.
file_path = os.path.join(directory, filename)
# Check if the file exists at the specified path.
return os.path.exists(file_path)
# Define a test case class 'TestFileExists' that inherits from 'unittest.TestCase'.
class TestFileExists(unittest.TestCase):
# Define a test method 'test_existing_file' to test the existence of an existing file.
def test_existing_file(self):
# Define the directory and filename for an existing file.
directory = '/path/txt'
filename = 'test1.txt'
# Assert that the file exists in the specified directory.
self.assertTrue(file_exists(directory, filename), "The file does not exist in the specified directory")
# Define a test method 'test_nonexistent_file' to test the non-existence of a non-existing file.
def test_nonexistent_file(self):
# Define the directory and filename for a non-existing file.
directory = '/path/txt'
filename = 'test2.txt'
# Assert that the file does not exist in the specified directory.
self.assertFalse(file_exists(directory, filename), "The file exists in the specified directory")
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
FAIL: test_existing_file (__main__.TestFileExists)
Ran 2 tests in 0.001s FAILED (failures=1)
Explanation:
In the above exercise,
- The function file_exists(directory, filename) takes a directory path and a filename as input and uses os.path.exists() to check if the file exists in the specified directory. It returns True if the file exists and False otherwise.
The TestFileExists class inherits from unittest.TestCase, and we define two test methods: test_existing_file() and test_nonexistent_file(). - In the test_existing_file() method, we provide the directory path and name of an existing file. We use the self.assertTrue() assertion to check if the file_exists() function returns True for this file.
- In the test_nonexistent_file() method, we provide the directory path and name of a non-existent file. We use the self.assertFalse() assertion to check if the file_exists() function returns False for this file.
Flowchart:
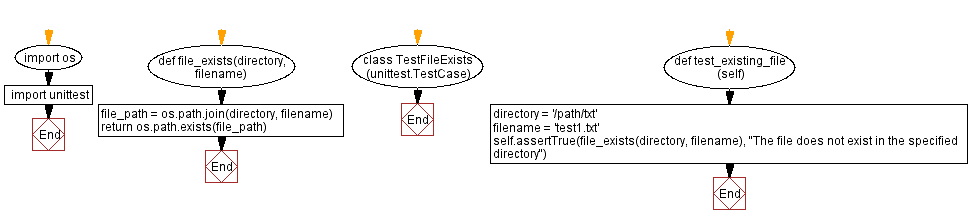
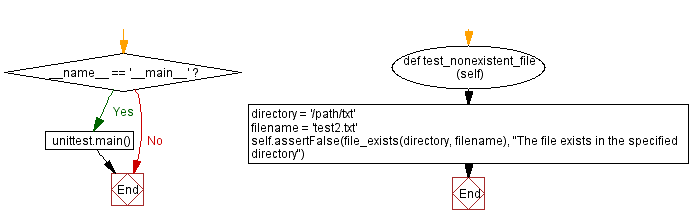
Previous: Check palindrome string.
Next: Accurate floating-point calculation handling.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics