Python file upload with urllib3: Making POST Requests simplified
Write a Python program that makes a POST request to upload a file to a server using urllib3.
Sample Solution:
Python Code :
import urllib3
def upload_file(url, file_path):
try:
# Create a PoolManager for managing the connection pool
http = urllib3.PoolManager()
# Open the file in binary mode for reading
with open(file_path, 'rb') as file:
# Read the content of the file
file_content = file.read()
# Make a POST request with the file content
response = http.request(
'POST',
url,
body=file_content,
headers={'Content-Type': 'application/octet-stream'}
)
# Check if the request was successful (status code 200)
if response.status == 200:
print("File upload successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to upload file. Status Code: {response.status}")
except Exception as e:
print(f"Error: {e}")
# Define the URL for file upload
upload_url = 'https://www.example.com/upload' # Replace with the actual upload URL
# Define the local file path to be uploaded
file_to_upload = 'test.txt' # Replace with the actual file path
# Make the POST request to upload the file
upload_file(upload_url, file_to_upload)
Sample Output:
Error: Unable to upload file. Status Code: 404
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Importing the urllib3 Module:
- Import the "urllib3" module, which is a powerful HTTP client for Python.
- Define the upload_file Function:
- Define a function named "upload_file()" that takes two parameters - 'url' (the URL for file upload) and 'file_path' (the local file path to be uploaded).
- Create a PoolManager:
- Creates a PoolManager object (http) to manage the connection pool for making HTTP requests.
- Open File in Binary Mode:
- Opens the file specified by 'file_path' in binary mode for reading ('rb').
- Read File Content:
- Reads the content of the file and stores it in the 'file_content' variable.
- Make a POST Request:
- Makes a POST request to the specified "url" with the file content as the request body.
- Sets the "Content-Type" header to indicate an octet-stream (binary) file.
- Check Response Status:
- Check if the response status code is 200.
- If successful, print a success message along with the response data.
- If unsuccessful, print an error message with the status code.
- Exception Handling:
- Handles any exceptions that might occur during the execution of the code and prints an error message.
- Defines Upload URL and Local File Path:
- Defines the URL for file upload ('upload_url') and the local file path to be uploaded ('file_to_upload').
- Makes the POST Request:
- Calls the "upload_file()" function with the specified upload URL and file path to initiate the file upload process.
Flowchart:
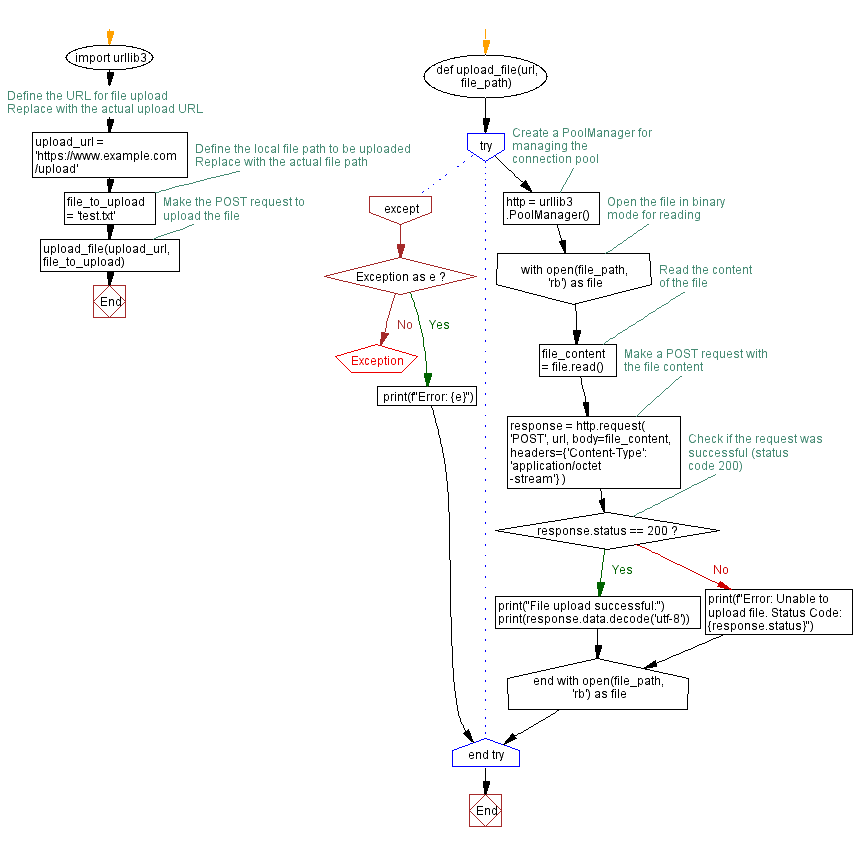
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Implementing Retry Mechanism in Python urllib3 for Resilient HTTP Requests.
Next: Python urllib3: Demonstrate Cookie persistence for seamless Web requests.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.