Python File Upload: Simulate POST request with multipart/Form-Data
Python urllib3 : Exercise-19 with Solution
Write a Python program that makes a POST request with multipart/form-data to simulate file uploads.
Sample Solution:
Python Code :
import urllib3
# Create a PoolManager
http = urllib3.PoolManager()
# Specify the file and URL
file_path = 'test.txt'
upload_url = 'https://example.com/upload'
# Prepare the multipart/form-data payload
fields = {
'field1': 'value1', # Additional form fields if needed
'field2': 'value2',
'file': ('filename.txt', open(file_path, 'rb').read(), 'text/plain')
}
# Set the Content-Type header to multipart/form-data
headers = {'Content-Type': 'multipart/form-data'}
# Make the POST request
response = http.request('POST', upload_url, fields=fields, headers=headers)
# Receive and print the response
print(response.data.decode('utf-8'))
Sample Output:
<?xml version="1.0" encoding="iso-8859-1"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <title>404 - Not Found</title> </head> <body> <h1>404 - Not Found</h1> <script type="text/javascript" src="//obj.ac.bcon.ecdns.net/ec_tpm_bcon.js"></script> </body> </html>
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import the necessary modules:
- Import the "urllib3" module.
- Create a PoolManager object:
- Create a PoolManager object to manage the connection pool for making HTTP requests.
- Specify the file and URL:
- Define the path to the file you want to upload.
- Specify the target URL for the file upload.
- Prepare the multipart/form-data Payload:
- Create a 'fields' dictionary to include form fields and the file to be uploaded.
- Set the 'Content-Type' header to 'multipart/form-data'.
- Make the POST Request:
- Use the "request()" method of the 'PoolManager' to send a POST request.
- Provide the URL, fields (payload), and headers.
- Receive and Print the Response:
- Receive the response and print the content
Flowchart:
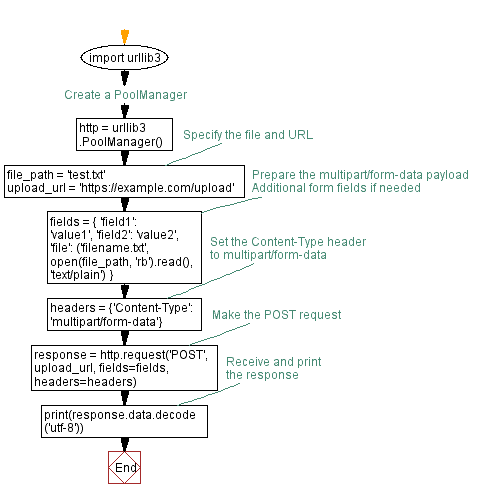
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python urllib3: Demonstrate Cookie persistence for seamless Web requests.
Next: Python Program: Maximum connection Pool size analysis.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics