Python Program: Custom Redirect Handling with urllib3
Python urllib3 : Exercise-22 with Solution
Write a Python program that implements custom logic to handle redirects based on specific conditions during a request.
Sample Solution:
Python Code :
import urllib3
# Custom redirect handling logic
def handle_redirect(response, redirect_location):
# Define custom logic to handle redirects based on specific conditions
if redirect_location.startswith('https://www.example.com'):
return True
return False
# Create a custom redirect pool manager
class CustomRedirectPoolManager(urllib3.PoolManager):
def redirect_request(self, method, url, **kwargs):
# Override redirect_request method to use custom redirect handling logic
response = super().request(method, url, **kwargs)
if response.status == 302 and 'Location' in response.headers:
redirect_location = response.headers['Location']
if handle_redirect(response, redirect_location):
return super().request(method, redirect_location, **kwargs)
return response
# Create a custom HTTP pool manager with the custom redirect adapter
http = CustomRedirectPoolManager()
# Define the URL for the initial request
initial_url = 'https://www.example.com'
try:
# Make a GET request
response = http.request('GET', initial_url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Request Successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except Exception as e:
print(f"Error: {e}")
Sample Output:
Request Successful: <!doctype html> <html> <head> <title>Example Domain</title> <meta charset="utf-8" /> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style type="text/css"> body { background-color: #f0f0f2; margin: 0; padding: 0; font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif; } div { width: 600px; margin: 5em auto; padding: 2em; background-color: #fdfdff; border-radius: 0.5em; box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02); } a:link, a:visited { color: #38488f; text-decoration: none; } @media (max-width: 700px) { div { margin: 0 auto; width: auto; } } </style> </head> <body> <div> <h1>Example Domain</h1> <p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p> <p><a href="https://www.iana.org/domains/example">More information...</a></p> </div> </body> </html>
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- We define a custom redirect pool manager "CustomRedirectPoolManager" by subclassing "urllib3.PoolManager".
- We override the "redirect_request()" method to use custom redirect handling logic defined in "handle_redirect".
- We define the "handle_redirect()" function to implement custom logic to handle redirects based on specific conditions.
- We create a custom HTTP pool manager 'http' using "CustomRedirectPoolManager".
- Finally, we make a GET request using the pool manager and handle the response accordingly.
Flowchart:
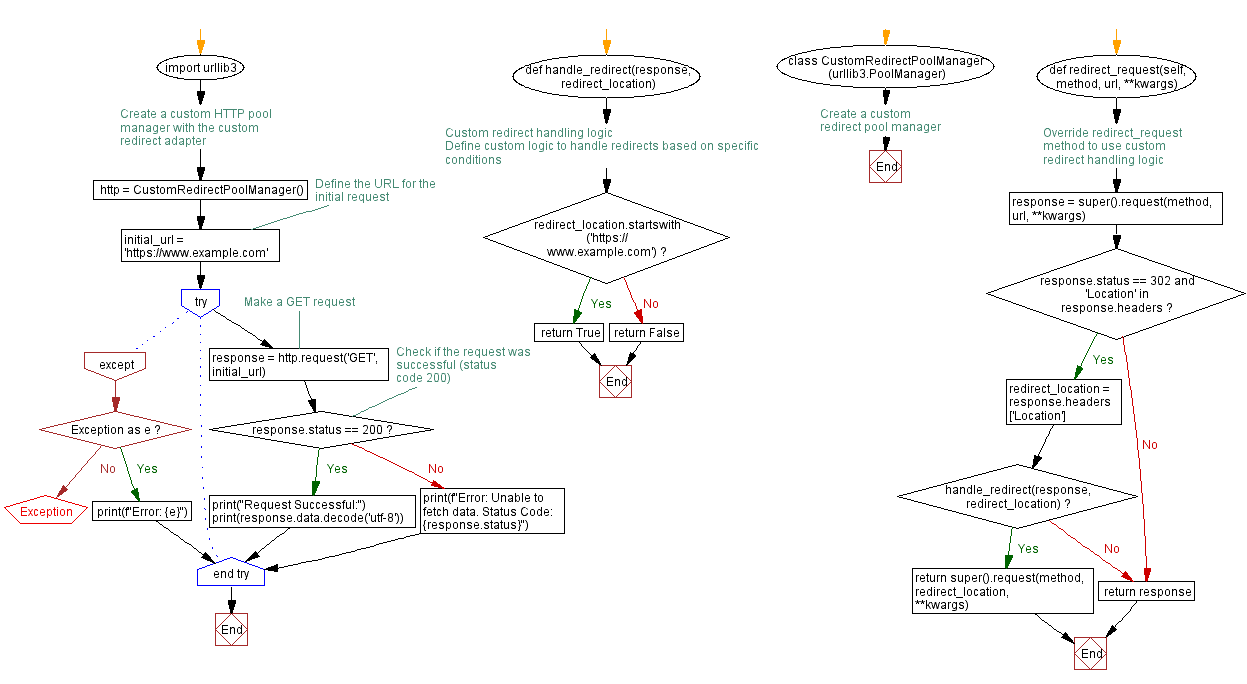
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Program: Observing urllib3 Response compression handling.
Next: Python Retry Mechanism with incremental timeouts.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics