Python Web Scraping: Find the live weather report of a given city
Write a Python program to find the live weather report (temperature, wind speed, description and weather) of a given city.
Sample Solution:
Python Code:
import requests
from pprint import pprint
def weather_data(query):
res=requests.get('http://api.openweathermap.org/data/2.5/weather?'+query+'&APPID=****************************8&units=metric');
return res.json();
def print_weather(result,city):
print("{}'s temperature: {}°C ".format(city,result['main']['temp']))
print("Wind speed: {} m/s".format(result['wind']['speed']))
print("Description: {}".format(result['weather'][0]['description']))
print("Weather: {}".format(result['weather'][0]['main']))
def main():
city=input('Enter the city:')
print()
try:
query='q='+city;
w_data=weather_data(query);
print_weather(w_data, city)
print()
except:
print('City name not found...')
if __name__=='__main__':
main()
Note: To create an Weather API from OpenWeather click here.
Sample Output:
Enter the city: Brazil Brazil's temperature: 16.45°C Wind speed: 2.1 m/s Description: clear sky Weather: Clear
Flowchart:
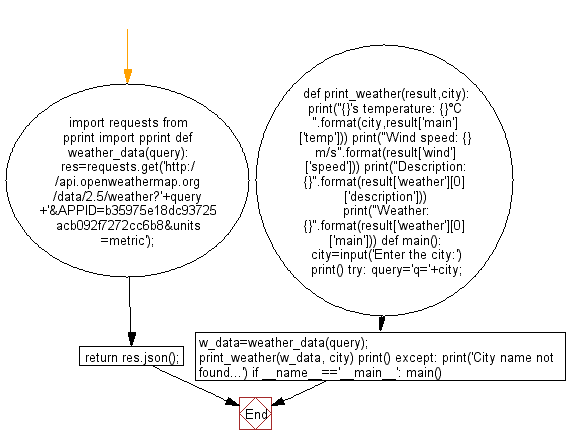
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to scrap number of tweets of a given Twitter account.
Next: Write a Python program to display the date, days, title, city, country of next 25 Hackevents.
What is the difficulty level of this exercise?