Python Web Scraping: Get movie name, year and a brief summary of the top 10 random movies
Python Web Scraping: Exercise-24 with Solution
Write a Python program to get movie name, year and a brief summary of the top 10 random movies.
Sample Solution:
Python Code:
from bs4 import BeautifulSoup
import requests
import random
def get_imd_movies(url):
page = requests.get(url)
soup = BeautifulSoup(page.text, 'html.parser')
movies = soup.find_all("td", class_="titleColumn")
random.shuffle(movies)
return movies
def get_imd_summary(url):
movie_page = requests.get(url)
soup = BeautifulSoup(movie_page.text, 'html.parser')
return soup.find("div", class_="summary_text").contents[0].strip()
def get_imd_movie_info(movie):
movie_title = movie.a.contents[0]
movie_year = movie.span.contents[0]
movie_url = 'http://www.imdb.com' + movie.a['href']
return movie_title, movie_year, movie_url
def imd_movie_picker():
ctr=0
print("--------------------------------------------")
for movie in get_imd_movies('http://www.imdb.com/chart/top'):
movie_title, movie_year, movie_url = get_imd_movie_info(movie)
movie_summary = get_imd_summary(movie_url)
print(movie_title, movie_year)
print(movie_summary)
print("--------------------------------------------")
ctr=ctr+1
if (ctr==10):
break;
if __name__ == '__main__':
imd_movie_picker()
Sample Output:
-------------------------------------------- Rashômon (1950) A heinous crime and its aftermath are recalled from differing points of view. -------------------------------------------- Raging Bull (1980) The life of boxer -------------------------------------------- Lawrence of Arabia (1962) The story of -------------------------------------------- La La Land (2016) While navigating their careers in Los Angeles, a pianist and an actress fall in love while attempting to reconcile their aspirations for the future. -------------------------------------------- The Help (2011) An aspiring author during the civil rights movement of the 1960s decides to write a book detailing the African American maids' point of view on the white families for which they work, and the hardships they go through on a daily basis. -------------------------------------------- The Godfather: Part II (1974) The early life and career of Vito Corleone in 1920s New York City is portrayed, while his son, Michael, expands and tightens his grip on the family crime syndicate. -------------------------------------------- Lock, Stock and Two Smoking Barrels (1998) A botched card game in London triggers four friends, thugs, weed-growers, hard gangsters, loan sharks and debt collectors to collide with each other in a series of unexpected events, all for the sake of weed, cash and two antique shotguns. -------------------------------------------- Wild Strawberries (1957) After living a life marked by coldness, an aging professor is forced to confront the emptiness of his existence. -------------------------------------------- M (1931) When the police in a German city are unable to catch a child-murderer, other criminals join in the manhunt. -------------------------------------------- The Seventh Seal (1957) A man seeks answers about life, death, and the existence of God as he plays chess against the Grim Reaper during the Black Plague. --------------------------------------------
Flowchart:
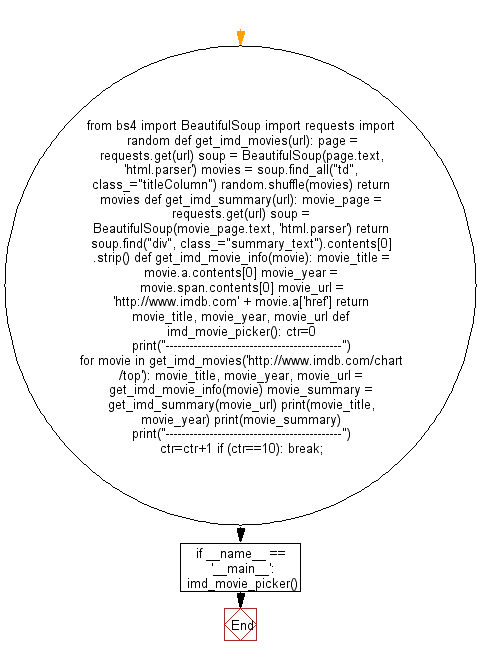
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to download IMDB's Top 250 data (movie name, Initial release, director name and stars).
Next: Write a Python program to get the number of magnitude 4.5+ earthquakes detected worldwide by the USGS.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics