Ruby Basic Exercises: Check three given integers and return their sum
Ruby Basic: Exercise-52 with Solution
Write a Ruby program to check three given integers and return their sum. However, If one of the values is the same as another of the values, it does not count towards the sum.
Ruby Code:
def check_num(a, b, c)
if a==b && b==c
return 0
elsif a==b
return c
elsif a==c
return b
elsif b==c
return a
else
return a+b+c
end
end
print check_num(5, 5, 5),"\n"
print check_num(5, 5, 7),"\n"
print check_num(5, 7, 5),"\n"
print check_num(7, 5, 5),"\n"
print check_num(1, 2, 3),"\n"
Output:
0 7 7 7 6
Flowchart:
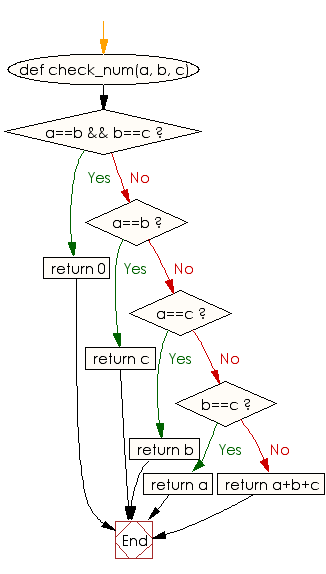
Ruby Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Ruby program to check two given integers, each in the range 10..99, return true if there is a digit that appears in both numbers.
Next: Write a Ruby program to check three given integers and compute their sum. However, if one of the values is 17 then it does not count towards the sum and values to its right do not count.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/ruby-exercises/basic/ruby-basic-exercise-52.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics