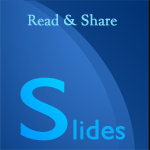
The art of command line
The art of command line.
Transcript
The art of command line
<!doctype html> <html lang="en"> <head> <title>The art of command line</title> </head> <body> </body> </html>
List of Contains
- Meta
- Basics
- Everyday use
- Processing files and data
- System debugging
- One-liners
- Obscure but useful
- OS X only
- Windows only
Meta
Scope:
- This guide is both for beginners and the experienced. The goals are breadth (everything important), specificity (give concrete examples of the most common case), and brevity (avoid things that aren't essential or digressions you can easily look up elsewhere). Every tip is essential in some situation or significantly saves time over alternatives.
- This is written for Linux, with the exception of the "OS X only" and "Windows only" sections. Many of the other items apply or can be installed on other Unices or OS X (or even Cygwin).
- The focus is on interactive Bash, though many tips apply to other shells and to general Bash scripting.
- It includes both "standard" Unix commands as well as ones that require special package installs -- so long as they are important enough to merit inclusion.
Notes:
- To keep this to one page, content is implicitly included by reference. You're smart enough to look up more detail elsewhere once you know the idea or command to Google. Use apt-get, yum, dnf, pacman, pip or brew (as appropriate) to install new programs.
- Use Explainshell to get a helpful breakdown of what commands, options, pipes etc. do.
Basics
- Learn basic Bash. Actually, type man bash and at least skim the whole thing; it's pretty easy to follow and not that long. Alternate shells can be nice, but Bash is powerful and always available (learning only zsh, fish, etc., while tempting on your own laptop, restricts you in many situations, such as using existing servers).
- Learn at least one text-based editor well. Ideally Vim (vi), as there's really no competition for random editing in a terminal (even if you use Emacs, a big IDE, or a modern hipster editor most of the time).
- Know how to read documentation with man (for the inquisitive, man man lists the section numbers, e.g. 1 is "regular" commands, 5 is files/conventions, and 8 are for administration). Find man pages with apropos. Know that some commands are not executables, but Bash builtins, and that you can get help on them with help and help -d.
- Learn about redirection of output and input using > and < and pipes using |. Know > overwrites the output file and >> appends. Learn about stdout and stderr.
- Learn about file glob expansion with * (and perhaps ? and [...]) and quoting and the difference between double " and single ' quotes. (See more on variable expansion below.)
- Be familiar with Bash job management: &, ctrl-z, ctrl-c, jobs, fg, bg, kill, etc.
- Know ssh, and the basics of passwordless authentication, via ssh-agent, ssh-add, etc.
- Basic file management: ls and ls -l (in particular, learn what every column in ls -l means), less, head, tail and tail -f (or even better, less +F), ln and ln -s (learn the differences and advantages of hard versus soft links), chown, chmod, du (for a quick summary of disk usage: du -hs *). For filesystem management, df, mount, fdisk, mkfs, lsblk. Learn what an inode is (ls -i or df -i).
- Basic network management: ip or ifconfig, dig.
- Learn and use a version control management system, such as git.
- Know regular expressions well, and the various flags to grep/egrep. The -i, -o, -v, -A, -B, and -C options are worth knowing.
- Learn to use apt-get, yum, dnf or pacman (depending on distro) to find and install packages. And make sure you have pip to install Python-based command-line tools (a few below are easiest to install via pip).
Everyday use
- In Bash, use Tab to complete arguments or list all available commands and ctrl-r to search through command history (after pressing, type to search, press ctrl-r repeatedly to cycle through more matches, press Enter to execute the found command, or hit the right arrow to put the result in the current line to allow editing).
- In Bash, use ctrl-w to delete the last word, and ctrl-u to delete all the way back to the start of the line. Use alt-b and alt-f to move by word, ctrl-a to move cursor to beginning of line, ctrl-e to move cursor to end of line, ctrl-k to kill to the end of the line, ctrl-l to clear the screen. See man readline for all the default keybindings in Bash. There are a lot. For example alt-. cycles through previous arguments, and alt-* expands a glob.
- Alternatively, if you love vi-style key-bindings, use set -o vi (and set -o emacs to put it back).
- For editing long commands, after setting your editor (for example export EDITOR=vim), ctrl-x ctrl-e will open the current command in an editor for multi-line editing. Or in vi style, escape-v.
- To see recent commands, history. There are also many abbreviations such as !$ (last argument) and !! last command, though these are often easily replaced with ctrl-r and alt-..
- Go to your home directory with cd. Access files relative to your home directory with the ~ prefix (e.g. ~/.bashrc). In sh scripts refer to the home directory as $HOME.
- To go back to the previous working directory: cd -.
- If you are halfway through typing a command but change your mind, hit alt-# to add a # at the beginning and enter it as a comment (or use ctrl-a, #, enter). You can then return to it later via command history.
- Use xargs (or parallel). It's very powerful. Note you can control how many items execute per line (-L) as well as parallelism (-P). If you're not sure if it'll do the right thing, use xargs echo first. Also, -I{} is handy.
Examples:
find . -name '*.py' | xargs grep some_function cat hosts | xargs -I{} ssh root@{} hostname
set -euo pipefail trap "echo 'error: Script failed: see failed command above'" ERR
# do something in current dir (cd /some/other/dir && other-command) # continue in original dir
diff /etc/hosts <(ssh somehost cat /etc/hosts)
TCPKeepAlive=yes ServerAliveInterval=15 ServerAliveCountMax=6 Compression=yes ControlMaster auto ControlPath /tmp/%r@%h:%p ControlPersist yes
stat -c '%A %a %n' /etc/timezone
>>> 2+3 5
Processing files and data
- To locate a file by name in the current directory, find . -iname '*something*' (or similar). To find a file anywhere by name, use locate something (but bear in mind updatedb may not have indexed recently created files).
- For general searching through source or data files (more advanced than grep -r), use ag.
- To convert HTML to text: lynx -dump -stdin
- For Markdown, HTML, and all kinds of document conversion, try pandoc.
- If you must handle XML, xmlstarlet is old but good.
- For JSON, use jq.
- For YAML, use shyaml.
- For Excel or CSV files, csvkit provides in2csv, csvcut, csvjoin, csvgrep, etc.
- For Amazon S3, s3cmd is convenient and s4cmd is faster. Amazon's aws and the improved saws are essential for other AWS-related tasks.
- Know about sort and uniq, including uniq's -u and -d options -- see one-liners below. See also comm.
- Know about cut, paste, and join to manipulate text files. Many people use cut but forget about join.
- Know about wc to count newlines (-l), characters (-m), words (-w) and bytes (-c).
- Know about tee to copy from stdin to a file and also to stdout, as in ls -al | tee file.txt.
- Know that locale affects a lot of command line tools in subtle ways, including sorting order (collation) and performance. Most Linux installations will set LANG or other locale variables to a local setting like US English. But be aware sorting will change if you change locale. And know i18n routines can make sort or other commands run many times slower. In some situations (such as the set operations or uniqueness operations below) you can safely ignore slow i18n routines entirely and use traditional byte-based sort order, using export LC_ALL=C.
- You can set a specific command's environment by prefixing its invocation with the environment variable settings, as in TZ=Pacific/Fiji date.
- Know basic awk and sed for simple data munging. For example, summing all numbers in the third column of a text file: awk '{ x += $3 } END { print x }'. This is probably 3X faster and 3X shorter than equivalent Python.
- To replace all occurrences of a string in place, in one or more files:
perl -pi.bak -e 's/old-string/new-string/g' my-files-*.txt
# Full rename of filenames, directories, and contents foo -> bar: repren --full --preserve-case --from foo --to bar . # Recover backup files whatever.bak -> whatever: repren --renames --from '(.*)\.bak' --to '\1' *.bak # Same as above, using rename, if available: rename 's/\.bak$//' *.bak
mkdir empty && rsync -r --delete empty/ some-dir && rmdir some-dir
uconv -f utf-8 -t utf-8 -x '::Any-Lower; ::Any-NFD; [:Nonspacing Mark:] >; ::Any-NFC; ' < input.txt > output.txt
For example:
getfacl -R /some/path > permissions.txt setfacl --restore=permissions.txt
System debugging
- For web debugging, curl and curl -I are handy, or their wget equivalents, or the more modern httpie.
- To know current cpu/disk status, the classic tools are top (or the better htop), iostat, and iotop. Use iostat -mxz 15 for basic CPU and detailed per-partition disk stats and performance insight.
- For network connection details, use netstat and ss.
- For a quick overview of what's happening on a system, dstat is especially useful. For broadest overview with details, use glances.
- To know memory status, run and understand the output of free and vmstat. In particular, be aware the "cached" value is memory held by the Linux kernel as file cache, so effectively counts toward the "free" value.
- Java system debugging is a different kettle of fish, but a simple trick on Oracle's and some other JVMs is that you can run kill -3 <pid> and a full stack trace and heap summary (including generational garbage collection details, which can be highly informative) will be dumped to stderr/logs. The JDK's jps, jstat, jstack, jmap are useful. SJK tools are more advanced.
- Use mtr as a better traceroute, to identify network issues.
- For looking at why a disk is full, ncdu saves time over the usual commands like du -sh *.
- To find which socket or process is using bandwidth, try iftop or nethogs.
- The ab tool (comes with Apache) is helpful for quick-and-dirty checking of web server performance. For more complex load testing, try siege.
- For more serious network debugging, wireshark, tshark, or ngrep.
- Know about strace and ltrace. These can be helpful if a program is failing, hanging, or crashing, and you don't know why, or if you want to get a general idea of performance. Note the profiling option (-c), and the ability to attach to a running process (-p).
- Know about ldd to check shared libraries etc.
- Know how to connect to a running process with gdb and get its stack traces.
- Use /proc. It's amazingly helpful sometimes when debugging live problems. Examples: /proc/cpuinfo, /proc/meminfo, /proc/cmdline, /proc/xxx/cwd, /proc/xxx/exe, /proc/xxx/fd/, /proc/xxx/smaps (where xxx is the process id or pid).
- When debugging why something went wrong in the past, sar can be very helpful. It shows historic statistics on CPU, memory, network, etc.
- For deeper systems and performance analyses, look at stap (SystemTap), perf, and sysdig.
- Check what OS you're on with uname or uname -a (general Unix/kernel info) or lsb_release -a (Linux distro info).
- Use dmesg whenever something's acting really funny (it could be hardware or driver issues).
- If you delete a file and it doesn't free up expected disk space as reported by du, check whether the file is in use by a process: lsof | grep deleted | grep "filename-of-my-big-file"
One-liners
A few examples of piecing together commands:
- It is remarkably helpful sometimes that you can do set intersection, union, and difference of text files via sort/uniq. Suppose a and b are text files that are already uniqued. This is fast, and works on files of arbitrary size, up to many gigabytes. (Sort is not limited by memory, though you may need to use the -T option if /tmp is on a small root partition.) See also the note about LC_ALL above and sort's -u option (left out for clarity below).
cat a b | sort | uniq > c # c is a union b cat a b | sort | uniq -d > c # c is a intersect b cat a b b | sort | uniq -u > c # c is set difference a - b
awk '{ x += $3 } END { print x }' myfile
find . -type f -ls
cat access.log | egrep -o 'acct_id=[0-9]+' | cut -d= -f2 | sort | uniq -c | sort -rn
function taocl() { curl -s https://raw.githubusercontent.com/jlevy/the-art-of-command-line/master/README.md | pandoc -f markdown -t html | xmlstarlet fo --html --dropdtd | xmlstarlet sel -t -v "(html/body/ul/li[count(p)>0])[$RANDOM mod last()+1]" | xmlstarlet unesc | fmt -80 }
Obscure but useful
- expr: perform arithmetic or boolean operations or evaluate regular expressions
- m4: simple macro processor
- yes: print a string a lot
- cal: nice calendar
- env: run a command (useful in scripts)
- printenv: print out environment variables (useful in debugging and scripts)
- look: find English words (or lines in a file) beginning with a string
- cut, paste and join: data manipulation
- fmt: format text paragraphs
- pr: format text into pages/columns
- fold: wrap lines of text
- column: format text fields into aligned, fixed-width columns or tables
- expand and unexpand: convert between tabs and spaces
- nl: add line numbers
- seq: print numbers
- bc: calculator
- factor: factor integers
- gpg: encrypt and sign files
- toe: table of terminfo entries
- nc: network debugging and data transfer
- socat: socket relay and tcp port forwarder (similar to netcat)
- slurm: network traffic visualization
- dd: moving data between files or devices
- file: identify type of a file
- tree: display directories and subdirectories as a nesting tree; like ls but recursive
- stat: file info
- time: execute and time a command
- timeout: execute a command for specified amount of time and stop the process when the specified amount of time completes.
- lockfile: create semaphore file that can only be removed by rm -f
- logrotate: rotate, compress and mail logs.
- watch: run a command repeatedly, showing results and/or highlighting changes
- tac: print files in reverse
- shuf: random selection of lines from a file
- comm: compare sorted files line by line
- pv: monitor the progress of data through a pipe
- hd, hexdump, xxd, biew and bvi: dump or edit binary files
- strings: extract text from binary files
- tr: character translation or manipulation
- iconv or uconv: conversion for text encodings
- split and csplit: splitting files
- sponge: read all input before writing it, useful for reading from then writing to the same file, e.g., grep -v something some-file | sponge some-file
- units: unit conversions and calculations; converts furlongs per fortnight to twips per blink (see also /usr/share/units/definitions.units)
- apg: generates random passwords
- 7z: high-ratio file compression
- ldd: dynamic library info
- nm: symbols from object files
- ab: benchmarking web servers
- strace: system call debugging
- mtr: better traceroute for network debugging
- cssh: visual concurrent shell
- rsync: sync files and folders over SSH or in local file system
- er SSH or in local file system
- wireshark and tshark: packet capture and network debugging
- ngrep: grep for the network layer
- host and dig: DNS lookups
- lsof: process file descriptor and socket info
- dstat: useful system stats
- glances: high level, multi-subsystem overview
- iostat: Disk usage stats
- mpstat: CPU usage stats
- vmstat: Memory usage stats
- htop: improved version of top
- last: login history
- w: who's logged on
- id: user/group identity info
- sar: historic system stats
- iftop or nethogs: network utilization by socket or process
- ss: socket statistics
- dmesg: boot and system error messages
- sysctl: view and configure Linux kernel parameters at run time
- hdparm: SATA/ATA disk manipulation/performance
- lsblk: list block devices: a tree view of your disks and disk partitions
- lshw, lscpu, lspci, lsusb, dmidecode: hardware information, including CPU, BIOS, RAID, graphics, devices, etc.
- lsmod and modinfo: List and show details of kernel modules.
- fortune, ddate, and sl: um, well, it depends on whether you consider steam locomotives and Zippy quotations "useful"
OS X only
These are items relevant only on OS X
- Package management with brew (Homebrew) and/or port (MacPorts). These can be used to install on OS X many of the above commands.
- Copy output of any command to a desktop app with pbcopy and paste input from one with pbpaste.
- To enable the Option key in OS X Terminal as an alt key (such as used in the commands above like alt-b, alt-f, etc.), open Preferences -> Profiles -> Keyboard and select "Use Option as Meta key".
- To open a file with a desktop app, use open or open -a /Applications/Whatever.app.
- Spotlight: Search files with mdfind and list metadata (such as photo EXIF info) with mdls.
- Be aware OS X is based on BSD Unix, and many commands (for example ps, ls, tail, awk, sed) have many subtle variations from Linux, which is largely influenced by System V-style Unix and GNU tools. You can often tell the difference by noting a man page has the heading "BSD General Commands Manual." In some cases GNU versions can be installed, too (such as gawk and gsed for GNU awk and sed). If writing cross-platform Bash scripts, avoid such commands (for example, consider Python or perl) or test carefully.
- To get OS X release information, use sw_vers.
Windows only
- Access the power of the Unix shell under Microsoft Windows by installing Cygwin. Most of the things described in this document will work out of the box.
- Install additional Unix programs with the Cygwin's package manager.
- Use mintty as your command-line window.
- Access the Windows clipboard through /dev/clipboard.
- Run cygstart to open an arbitrary file through its registered application.
- Access the Windows registry with regtool.
- Note that a C:\ Windows drive path becomes /cygdrive/c under Cygwin, and that Cygwin's / appears under C:\cygwin on Windows. Convert between Cygwin and Windows-style file paths with cygpath. This is most useful in scripts that invoke Windows programs.
- You can perform and script most Windows system administration tasks from the command line by learning and using wmic.