SQL Exercise: Names of patients, their doctors, and medications
19. From the following tables, write a SQL query to locate the patients' treating physicians and medications. Return Patient name as "Patient", Physician name as "Physician", Medication name as "Medication".
Sample table: patientssn | name | address | phone | insuranceid | pcp -----------+-------------------+--------------------+----------+-------------+----- 100000001 | John Smith | 42 Foobar Lane | 555-0256 | 68476213 | 1 100000002 | Grace Ritchie | 37 Snafu Drive | 555-0512 | 36546321 | 2 100000003 | Random J. Patient | 101 Omgbbq Street | 555-1204 | 65465421 | 2 100000004 | Dennis Doe | 1100 Foobaz Avenue | 555-2048 | 68421879 | 3Sample table: prescribes
physician | patient | medication | date | appointment | dose -----------+-----------+------------+---------------------+-------------+------ 1 | 100000001 | 1 | 2008-04-24 10:47:00 | 13216584 | 5 9 | 100000004 | 2 | 2008-04-27 10:53:00 | 86213939 | 10 9 | 100000004 | 2 | 2008-04-30 16:53:00 | | 5Sample table: physician
employeeid | name | position | ssn ------------+-------------------+------------------------------+----------- 1 | John Dorian | Staff Internist | 111111111 2 | Elliot Reid | Attending Physician | 222222222 3 | Christopher Turk | Surgical Attending Physician | 333333333 4 | Percival Cox | Senior Attending Physician | 444444444 5 | Bob Kelso | Head Chief of Medicine | 555555555 6 | Todd Quinlan | Surgical Attending Physician | 666666666 7 | John Wen | Surgical Attending Physician | 777777777 8 | Keith Dudemeister | MD Resident | 888888888 9 | Molly Clock | Attending Psychiatrist | 999999999Sample table: medication
code | name | brand | description ------+--------------+-----------------------+------------- 1 | Procrastin-X | X | N/A 2 | Thesisin | Foo Labs | N/A 3 | Awakin | Bar Laboratories | N/A 4 | Crescavitin | Baz Industries | N/A 5 | Melioraurin | Snafu Pharmaceuticals | N/A
Sample Solution:
-- SELECTing patient name, physician name, and medication name using INNER JOINs and WHERE clause
SELECT t.name AS "Patient",
p.name AS "Physician",
m.name AS "Medication"
-- FROM patient table aliased as t
FROM patient t
-- INNER JOIN with prescribes table aliased as s based on patient's SSN
JOIN prescribes s ON s.patient = t.ssn
-- INNER JOIN with physician table aliased as p based on physician's employeeid
JOIN physician p ON s.physician = p.employeeid
-- INNER JOIN with medication table aliased as m based on medication code
JOIN medication m ON s.medication = m.code
-- WHERE clause to filter results based on specific conditions if needed
-- Example: WHERE p.specialty = 'Cardiology';
Sample Output:
Patient | Physician | Medication ------------+-------------+-------------- John Smith | John Dorian | Procrastin-X Dennis Doe | Molly Clock | Thesisin Dennis Doe | Molly Clock | Thesisin (3 rows)
Explanation:
The said query in SQL that returns information about medications prescribed to patients by their physicians, including the patient's name, the physician's name, and the medication name.
The query performs a join between the 'patient', 'prescribes', 'physician', and 'medication' tables, based on their relational columns.
The 'prescribes' and 'patient' tables are joins based on the patient and ssn columns, the 'prescribes' and 'physician tables are joins based on the physician and employeeid columns, and the 'medication ' and the 'prescribes' and 'medication' tables are joins based on the medication and code columns.
Alternative Solution:
Using Implicit INNER JOIN:
-- SELECTing patient name, physician name, and medication name using implicit INNER JOINs
SELECT t.name AS "Patient",
p.name AS "Physician",
m.name AS "Medication"
-- FROM patient table aliased as t, prescribes table aliased as s, physician table aliased as p, medication table aliased as m
FROM patient t, prescribes s, physician p, medication m
-- WHERE clause specifying join conditions
WHERE s.patient = t.ssn
AND s.physician = p.employeeid
AND s.medication = m.code;
Explanation:
This solution uses the traditional comma-separated table list in the FROM clause with join conditions specified in the WHERE clause, creating implicit INNER JOINs.
Practice Online
E R Diagram of Hospital Database:
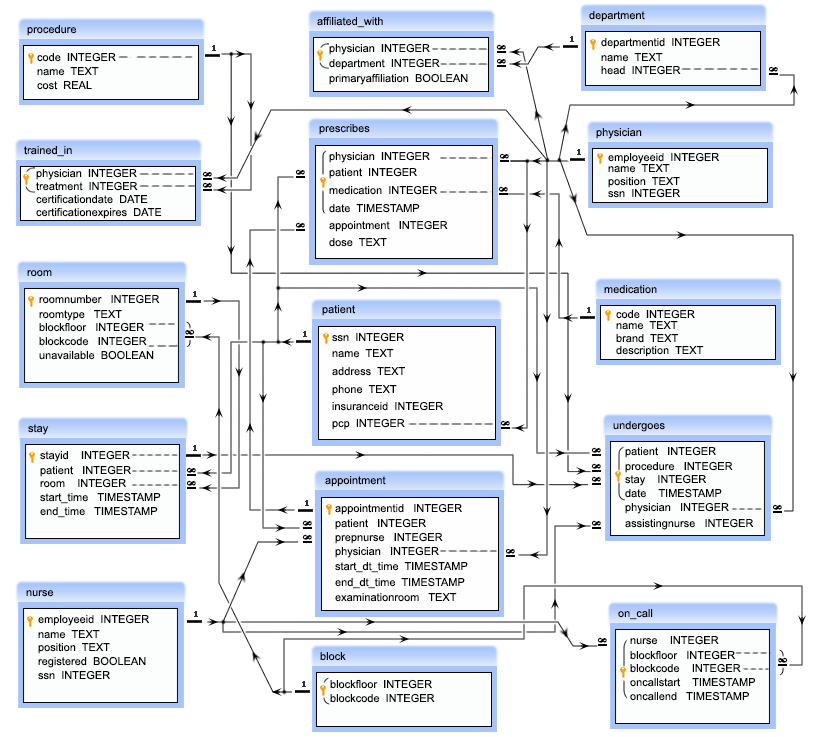
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Patients and their physicians who do not need a nurse.
Next SQL Exercise: Patients with their doctors and medications.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.