SQL Exercise: Physicians, a medical procedure without certification
31. From the following tables, write a SQL query to find all physicians who have performed a medical procedure but are not certified to do so. Return Physician name as "Physician".
Sample table: physicianemployeeid | name | position | ssn ------------+-------------------+------------------------------+----------- 1 | John Dorian | Staff Internist | 111111111 2 | Elliot Reid | Attending Physician | 222222222 3 | Christopher Turk | Surgical Attending Physician | 333333333 4 | Percival Cox | Senior Attending Physician | 444444444 5 | Bob Kelso | Head Chief of Medicine | 555555555 6 | Todd Quinlan | Surgical Attending Physician | 666666666 7 | John Wen | Surgical Attending Physician | 777777777 8 | Keith Dudemeister | MD Resident | 888888888 9 | Molly Clock | Attending Psychiatrist | 999999999Sample table: undergoes
physician | treatment | certificationdate | certificationexpires -----------+-----------+-------------------+---------------------- 3 | 1 | 2008-01-01 | 2008-12-31 3 | 2 | 2008-01-01 | 2008-12-31 3 | 5 | 2008-01-01 | 2008-12-31 3 | 6 | 2008-01-01 | 2008-12-31 3 | 7 | 2008-01-01 | 2008-12-31 6 | 2 | 2008-01-01 | 2008-12-31 6 | 5 | 2007-01-01 | 2007-12-31 6 | 6 | 2008-01-01 | 2008-12-31 7 | 1 | 2008-01-01 | 2008-12-31 7 | 2 | 2008-01-01 | 2008-12-31 7 | 3 | 2008-01-01 | 2008-12-31 7 | 4 | 2008-01-01 | 2008-12-31 7 | 5 | 2008-01-01 | 2008-12-31 7 | 6 | 2008-01-01 | 2008-12-31 7 | 7 | 2008-01-01 | 2008-12-31Sample table: trained_in
physician | treatment | certificationdate | certificationexpires -----------+-----------+-------------------+---------------------- 3 | 1 | 2008-01-01 | 2008-12-31 3 | 2 | 2008-01-01 | 2008-12-31 3 | 5 | 2008-01-01 | 2008-12-31 3 | 6 | 2008-01-01 | 2008-12-31 3 | 7 | 2008-01-01 | 2008-12-31 6 | 2 | 2008-01-01 | 2008-12-31 6 | 5 | 2007-01-01 | 2007-12-31 6 | 6 | 2008-01-01 | 2008-12-31 7 | 1 | 2008-01-01 | 2008-12-31 7 | 2 | 2008-01-01 | 2008-12-31 7 | 3 | 2008-01-01 | 2008-12-31 7 | 4 | 2008-01-01 | 2008-12-31 7 | 5 | 2008-01-01 | 2008-12-31 7 | 6 | 2008-01-01 | 2008-12-31 7 | 7 | 2008-01-01 | 2008-12-31
Sample Solution:
SELECT name AS "Physician"
FROM physician
WHERE employeeid IN
( SELECT undergoes.physician
FROM undergoes
LEFT JOIN trained_In ON undergoes.physician=trained_in.physician
AND undergoes.procedure=trained_in.treatment
WHERE treatment IS NULL );
Sample Output:
Physician ------------------ Christopher Turk (1 row)
Explanation:
The said query in SQL retrieves the name of physicians who have not been trained in a particular medical procedure by looking for records in the 'undergoes' table where a corresponding record in the 'trained_in' table with the same physician and procedure does not exist.
From the outer query filters the results by selecting only those records where the "employeeid" column is found in a subquery.
In the subquery the LEFT JOIN keyword joins the 'undergoes' and 'trained_in' tables based on the common columns physician, procedure, and treatment.
The WHERE clause in the subquery filters the results of the join to include only those records where "treatment" is NULL.
Practice Online
E R Diagram of Hospital Database:
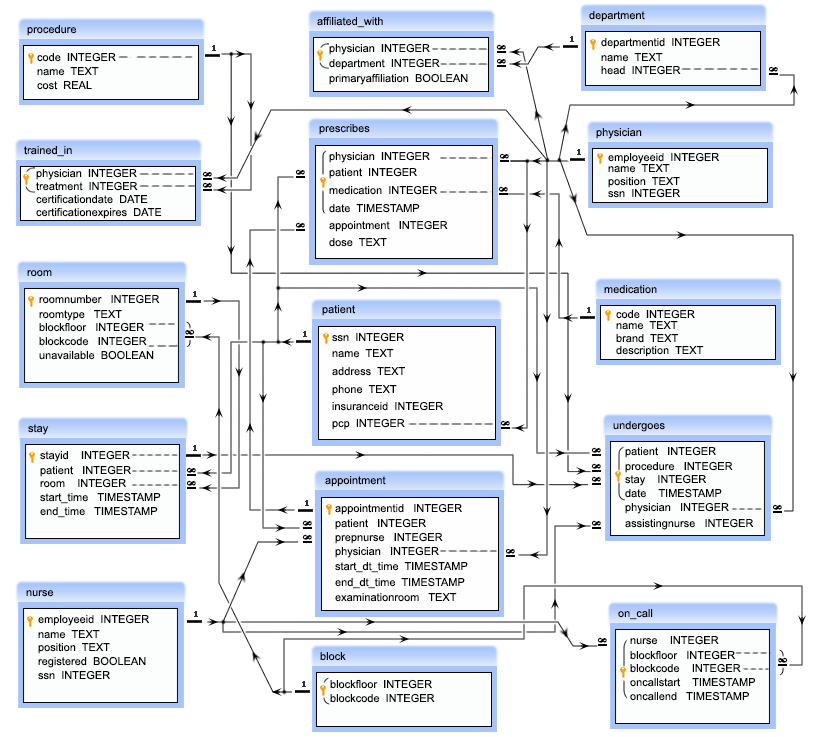
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Make a report of specified queries.
Next SQL Exercise: Doctors do the same procedure but are not certified.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.