SQL Exercise: Find all physicians who completed a medical procedure
33. From the following table, write a SQL query to find all physicians who completed a medical procedure with certification after the expiration date of their license. Return Physician Name as "Physician", Position as "Position".
Sample table: physicianemployeeid | name | position | ssn ------------+-------------------+------------------------------+----------- 1 | John Dorian | Staff Internist | 111111111 2 | Elliot Reid | Attending Physician | 222222222 3 | Christopher Turk | Surgical Attending Physician | 333333333 4 | Percival Cox | Senior Attending Physician | 444444444 5 | Bob Kelso | Head Chief of Medicine | 555555555 6 | Todd Quinlan | Surgical Attending Physician | 666666666 7 | John Wen | Surgical Attending Physician | 777777777 8 | Keith Dudemeister | MD Resident | 888888888 9 | Molly Clock | Attending Psychiatrist | 999999999Sample table: undergoes
physician | treatment | certificationdate | certificationexpires -----------+-----------+-------------------+---------------------- 3 | 1 | 2008-01-01 | 2008-12-31 3 | 2 | 2008-01-01 | 2008-12-31 3 | 5 | 2008-01-01 | 2008-12-31 3 | 6 | 2008-01-01 | 2008-12-31 3 | 7 | 2008-01-01 | 2008-12-31 6 | 2 | 2008-01-01 | 2008-12-31 6 | 5 | 2007-01-01 | 2007-12-31 6 | 6 | 2008-01-01 | 2008-12-31 7 | 1 | 2008-01-01 | 2008-12-31 7 | 2 | 2008-01-01 | 2008-12-31 7 | 3 | 2008-01-01 | 2008-12-31 7 | 4 | 2008-01-01 | 2008-12-31 7 | 5 | 2008-01-01 | 2008-12-31 7 | 6 | 2008-01-01 | 2008-12-31 7 | 7 | 2008-01-01 | 2008-12-31Sample table: trained_in
physician | treatment | certificationdate | certificationexpires -----------+-----------+-------------------+---------------------- 3 | 1 | 2008-01-01 | 2008-12-31 3 | 2 | 2008-01-01 | 2008-12-31 3 | 5 | 2008-01-01 | 2008-12-31 3 | 6 | 2008-01-01 | 2008-12-31 3 | 7 | 2008-01-01 | 2008-12-31 6 | 2 | 2008-01-01 | 2008-12-31 6 | 5 | 2007-01-01 | 2007-12-31 6 | 6 | 2008-01-01 | 2008-12-31 7 | 1 | 2008-01-01 | 2008-12-31 7 | 2 | 2008-01-01 | 2008-12-31 7 | 3 | 2008-01-01 | 2008-12-31 7 | 4 | 2008-01-01 | 2008-12-31 7 | 5 | 2008-01-01 | 2008-12-31 7 | 6 | 2008-01-01 | 2008-12-31 7 | 7 | 2008-01-01 | 2008-12-31
Sample Solution:
SELECT name AS "Physician",
position AS "Position"
FROM physician
WHERE employeeid IN
( SELECT physician
FROM undergoes u
WHERE date >
( SELECT certificationexpires
FROM trained_in t
WHERE t.physician = u.physician
AND t.treatment = u.procedure ) );
Sample Output:
Physician | Position --------------+------------------------------ Todd Quinlan | Surgical Attending Physician (1 row)
Explanation:
This SQL query retrieves the names and positions of physicians whose certification has expired for a procedure they have performed.
The WHERE clause filters the results where the "employeeid" column is found in a subquery.
The WHERE clause of the subquery include only those records where the "date" column is greater than the certification expiration date for the procedure.
The certification expiration date is obtained from inner most subquery which selects the "certificationexpires" column from the 'trained_in' table where the physician and procedure match those in the outer subquery.
Practice Online
E R Diagram of Hospital Database:
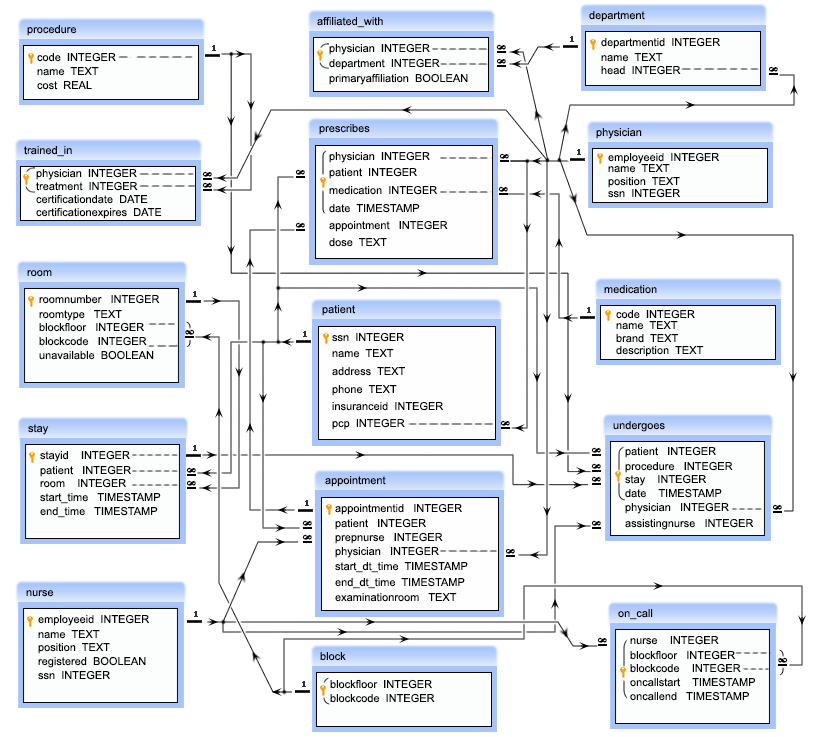
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Doctors do the same procedure but are not certified.
Next SQL Exercise: Medical procedures done after certification expired.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.