SQL exercises on movie Database: Find the movie title, and the highest number of stars that movie received
11. From the following tables, write a SQL query to find those movies, which have received highest number of stars. Group the result set on movie title and sorts the result-set in ascending order by movie title. Return movie title and maximum number of review stars.
Sample table: ratingmov_id | rev_id | rev_stars | num_o_ratings --------+--------+-----------+--------------- 901 | 9001 | 8.40 | 263575 902 | 9002 | 7.90 | 20207 903 | 9003 | 8.30 | 202778 906 | 9005 | 8.20 | 484746 924 | 9006 | 7.30 | 908 | 9007 | 8.60 | 779489 909 | 9008 | | 227235 910 | 9009 | 3.00 | 195961 911 | 9010 | 8.10 | 203875 912 | 9011 | 8.40 | 914 | 9013 | 7.00 | 862618 915 | 9001 | 7.70 | 830095 916 | 9014 | 4.00 | 642132 925 | 9015 | 7.70 | 81328 918 | 9016 | | 580301 920 | 9017 | 8.10 | 609451 921 | 9018 | 8.00 | 667758 922 | 9019 | 8.40 | 511613 923 | 9020 | 6.70 | 13091Sample table: movie
mov_id | mov_title | mov_year | mov_time | mov_lang | mov_dt_rel | mov_rel_country --------+----------------------------------------------------+----------+----------+-----------------+------------+----------------- 901 | Vertigo | 1958 | 128 | English | 1958-08-24 | UK 902 | The Innocents | 1961 | 100 | English | 1962-02-19 | SW 903 | Lawrence of Arabia | 1962 | 216 | English | 1962-12-11 | UK 904 | The Deer Hunter | 1978 | 183 | English | 1979-03-08 | UK 905 | Amadeus | 1984 | 160 | English | 1985-01-07 | UK 906 | Blade Runner | 1982 | 117 | English | 1982-09-09 | UK 907 | Eyes Wide Shut | 1999 | 159 | English | | UK 908 | The Usual Suspects | 1995 | 106 | English | 1995-08-25 | UK 909 | Chinatown | 1974 | 130 | English | 1974-08-09 | UK 910 | Boogie Nights | 1997 | 155 | English | 1998-02-16 | UK 911 | Annie Hall | 1977 | 93 | English | 1977-04-20 | USA 912 | Princess Mononoke | 1997 | 134 | Japanese | 2001-10-19 | UK 913 | The Shawshank Redemption | 1994 | 142 | English | 1995-02-17 | UK 914 | American Beauty | 1999 | 122 | English | | UK 915 | Titanic | 1997 | 194 | English | 1998-01-23 | UK 916 | Good Will Hunting | 1997 | 126 | English | 1998-06-03 | UK 917 | Deliverance | 1972 | 109 | English | 1982-10-05 | UK 918 | Trainspotting | 1996 | 94 | English | 1996-02-23 | UK 919 | The Prestige | 2006 | 130 | English | 2006-11-10 | UK 920 | Donnie Darko | 2001 | 113 | English | | UK 921 | Slumdog Millionaire | 2008 | 120 | English | 2009-01-09 | UK 922 | Aliens | 1986 | 137 | English | 1986-08-29 | UK 923 | Beyond the Sea | 2004 | 118 | English | 2004-11-26 | UK 924 | Avatar | 2009 | 162 | English | 2009-12-17 | UK 926 | Seven Samurai | 1954 | 207 | Japanese | 1954-04-26 | JP 927 | Spirited Away | 2001 | 125 | Japanese | 2003-09-12 | UK 928 | Back to the Future | 1985 | 116 | English | 1985-12-04 | UK 925 | Braveheart | 1995 | 178 | English | 1995-09-08 | UK
Sample Solution:
-- Selecting movie title and maximum review stars
-- Using the 'movie' and 'rating' tables
-- Joining tables based on mov_id
-- Filtering out rows where rev_stars is not NULL
SELECT mov_title, MAX(rev_stars)
FROM movie, rating
WHERE movie.mov_id = rating.mov_id
AND rating.rev_stars IS NOT NULL
-- Grouping the result set by mov_title
GROUP BY mov_title
-- Sorting the result set by mov_title
ORDER BY mov_title;
Sample Output:
mov_title | max ----------------------------------------------------+------ Aliens | 8.40 American Beauty | 7.00 Annie Hall | 8.10 Avatar | 7.30 Beyond the Sea | 6.70 Blade Runner | 8.20 Boogie Nights | 3.00 Braveheart | 7.70 Donnie Darko | 8.10 Good Will Hunting | 4.00 Lawrence of Arabia | 8.30 Princess Mononoke | 8.40 Slumdog Millionaire | 8.00 The Innocents | 7.90 The Usual Suspects | 8.60 Titanic | 7.70 Vertigo | 8.40 (17 rows)
Code Explanation:
The said query in SQL that retrieves the movie titles along with the maximum rating stars for each movie, where the ratings are not null. The results are sorted in alphabetical order based on the movie titles.
The query joins the movie and rating tables using the mov_id column, then applies a filter to exclude any rows where the rev_stars column is null. The MAX function is used to retrieve the highest rating value for each movie title, and the GROUP BY clause is used to group the results by mov_title. The ORDER BY clause sorts the results in alphabetical order based on mov_title.
Alternative Solution:
Using INNER JOIN and MAX() Aggregate Function:
SELECT m.mov_title, MAX(r.rev_stars) AS max_rev_stars
FROM movie m
JOIN rating r ON m.mov_id = r.mov_id
WHERE r.rev_stars IS NOT NULL
GROUP BY m.mov_title
ORDER BY m.mov_title;
Explanation:
This query employs an INNER JOIN to combine the movie and rating tables based on mov_id. It then uses the MAX() aggregate function to find the maximum rev_stars for each movie. The WHERE clause filters out rows with null rev_stars.
Relational Algebra Expression:
Relational Algebra Tree:
Practice Online
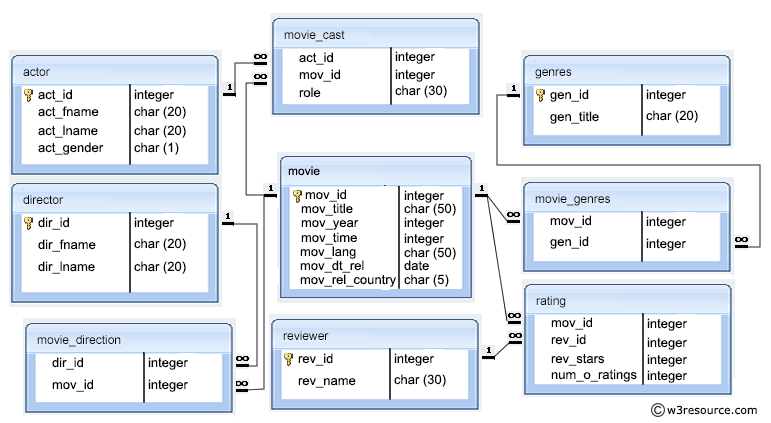
Query Visualization:
Duration:
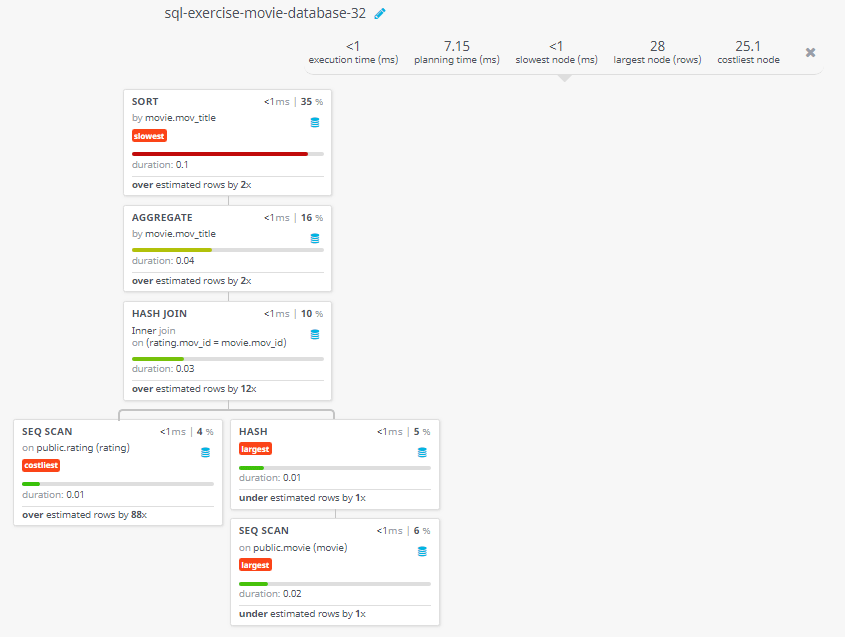
Rows:
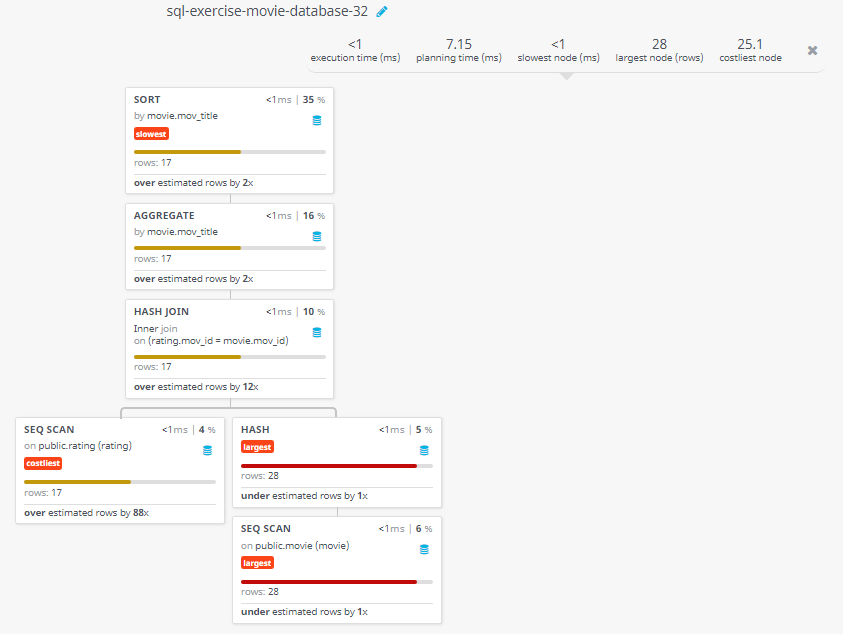
Cost:
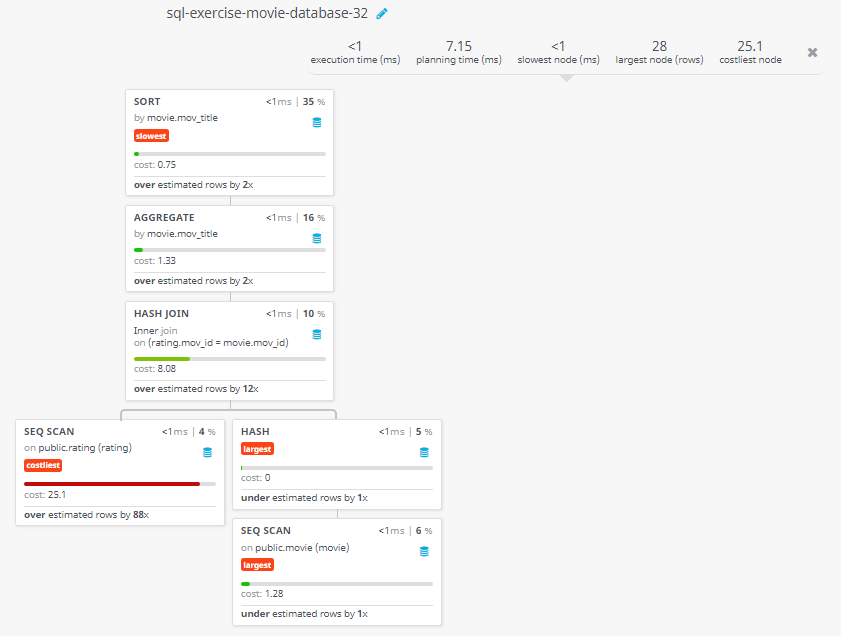
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: From the following tables, write a SQL query to find those reviewers who rated more than one movie. Group the result set on reviewer’s name, movie title. Return reviewer’s name, movie title.
Next: From the following tables, write a SQL query to find all reviewers who rated the movie ‘American Beauty’. Return reviewer name.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.