SQL Exercise: Customer who is neither in the list nor have a grade
16. Customer Order & Grade Report
Write a SQL statement to generate a report with the customer name, city, order no. order date, purchase amount for only those customers on the list who must have a grade and placed one or more orders or which order(s) have been placed by the customer who neither is on the list nor has a grade.
Sample table: customer
customer_id | cust_name | city | grade | salesman_id -------------+----------------+------------+-------+------------- 3002 | Nick Rimando | New York | 100 | 5001 3007 | Brad Davis | New York | 200 | 5001 3005 | Graham Zusi | California | 200 | 5002 3008 | Julian Green | London | 300 | 5002 3004 | Fabian Johnson | Paris | 300 | 5006 3009 | Geoff Cameron | Berlin | 100 | 5003 3003 | Jozy Altidor | Moscow | 200 | 5007 3001 | Brad Guzan | London | | 5005
Sample table: orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample Solution:
-- Selecting specific columns and renaming one column for clarity
SELECT a.cust_name, a.city, b.ord_no,
b.ord_date, b.purch_amt AS "Order Amount"
-- Specifying the tables to retrieve data from ('customer' as 'a' and 'orders' as 'b')
FROM customer a
-- Performing a full outer join based on the customer_id, including unmatched rows from both 'customer' and 'orders'
FULL OUTER JOIN orders b
ON a.customer_id = b.customer_id
-- Filtering the results based on the condition that grade is not null in 'customer'
WHERE a.grade IS NOT NULL;
Output of the Query:
cust_name city ord_no ord_date Order Amount Brad Guzan London 70009 2012-09-10 270.65 Nick Rimando New York 70002 2012-10-05 65.26 Geoff Cameron Berlin 70004 2012-08-17 110.50 Brad Davis New York 70005 2012-07-27 2400.60 Nick Rimando New York 70008 2012-09-10 5760.00 Fabian Johnson Paris 70010 2012-10-10 1983.43 Geoff Cameron Berlin 70003 2012-10-10 2480.40 Jozy Altidor Moscow 70011 2012-08-17 75.29 Nick Rimando New York 70013 2012-04-25 3045.60 Graham Zusi California 70001 2012-10-05 150.50 Graham Zusi California 70007 2012-09-10 948.50 Julian Green London 70012 2012-06-27 250.45
Explanation:
The said SQL query that is joining two tables: customer alias a, and orders alias b. The query is using a FULL OUTER JOIN on customer and orders tables on the 'customer_id' column.
The query is then selecting several columns from each table: 'cust_name' and 'city' from customer table, 'ord_no', 'ord_date', and 'purch_amt' aliased as 'Order Amount' from orders table.
The FULL OUTER JOIN ensures that all rows from both the customer table and the orders table are included in the result set, with NULL values in the columns of the table that doesn't have any matching rows for a customer.
The query also filters the results based on the condition "a.grade IS NOT NULL", which means it will only return the rows where the grade of the customer is not null. This means that rows where the customer doesn't have any grade will not be included in the result set.
Visual Explanation:
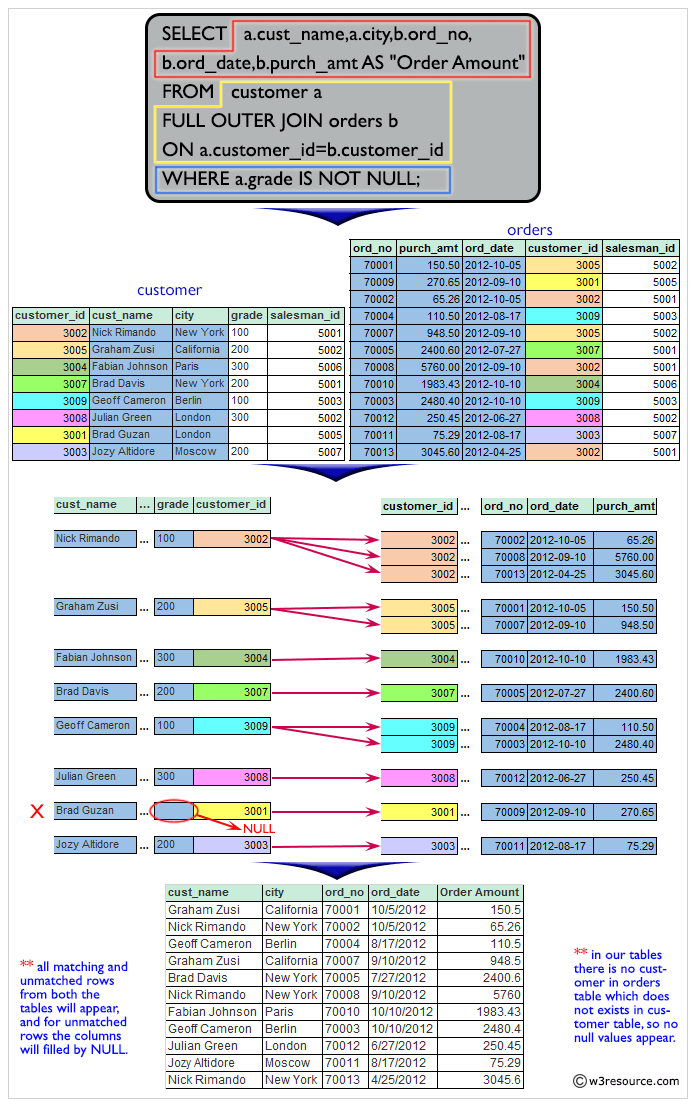
N.B.: In certain instances not null is removed in table structure, so results may vary.
Practice Online
Query Visualization:
Duration:
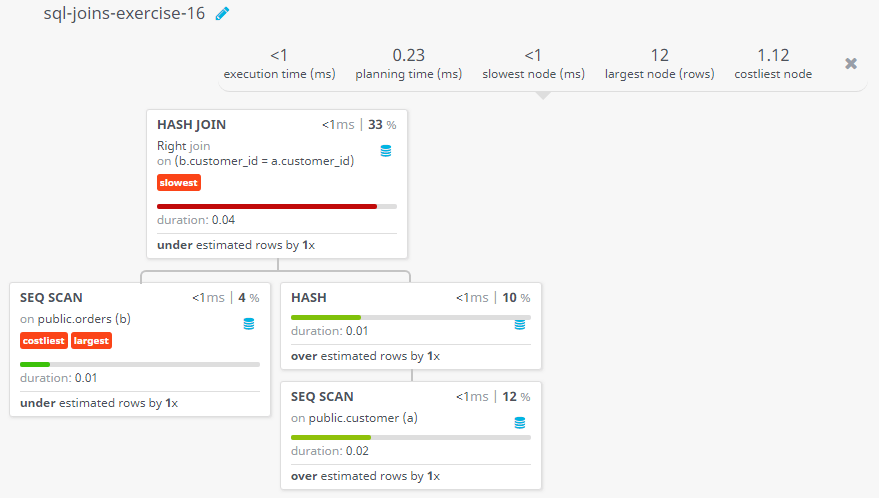
Rows:
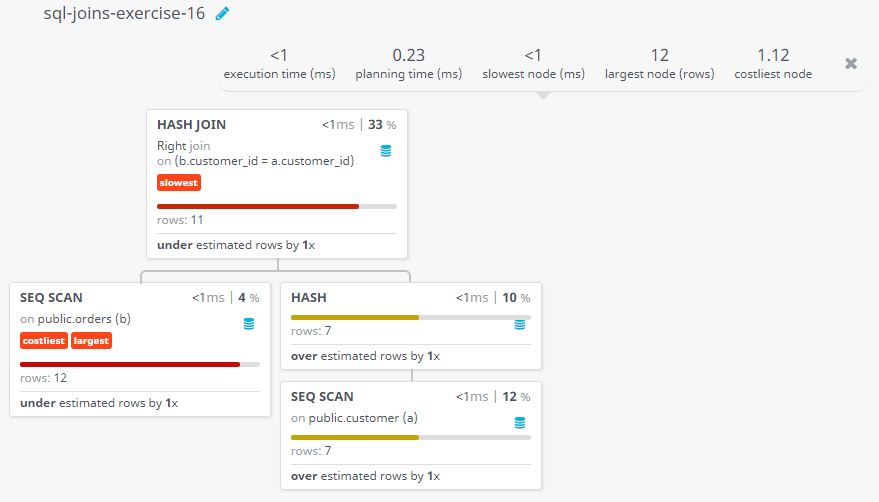
Cost:
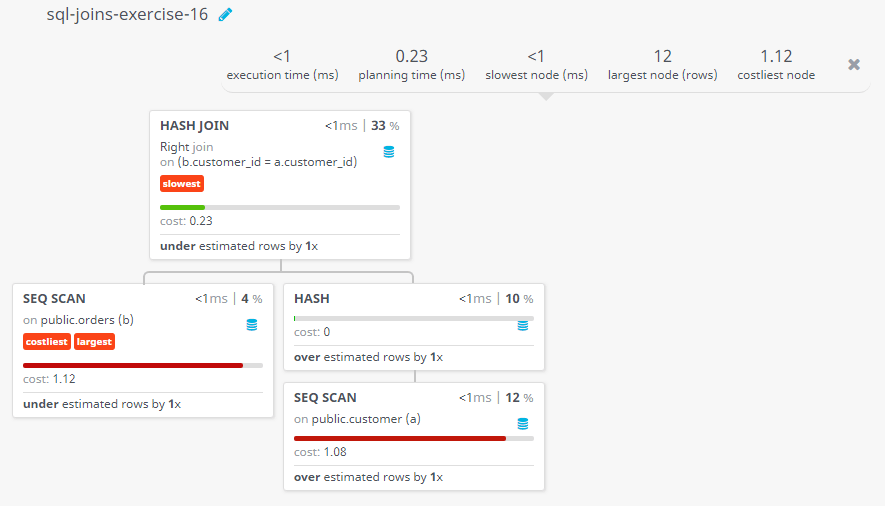
For more Practice: Solve these Related Problems:
- Write a SQL query to generate a report listing customer name, city, order number, order date, and purchase amount for customers with a non NULL grade who have placed at least one order, using an INNER JOIN.
- Write a SQL query to combine two result sets—one for customers with a grade who placed orders and one for customers without a grade who still placed orders—using UNION.
- Write a SQL query to display order details for customers, applying a LEFT JOIN on orders and filtering with a CASE statement to include only those with a grade or with orders despite a missing grade.
- Write a SQL query to produce a report of customer orders that uses a subquery to check for the existence of orders and a condition on the grade, then combines the results with a UNION ALL.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: One or more customers ordered from the existing list
Next SQL Exercise: Salesman will appear for all customer and vice versa.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.