Swift Array Programming Exercises: Test if a given array of integers contains three increasing adjacent numbers
Write a Swift program to test if a given array of integers contains three increasing adjacent numbers.
Example: a) 3, 4, 5 b) 7, 8, 9
Pictorial Presentation:
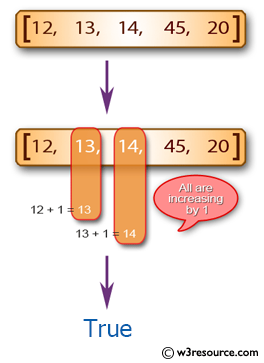
Sample Solution:
Swift Code:
func adjacent_numbers(array_nums: [Int]) -> Bool {
for x in 0..<array_nums.count-2 {
if array_nums[x] == array_nums[x+1] - 1 && array_nums[x+1] == array_nums[x+2] - 1
{
return true
}
}
return false
}
print(adjacent_numbers(array_nums: [1, 4, 5, 6, 2]))
print(adjacent_numbers(array_nums: [1, 2, 7, 4, 5]))
print(adjacent_numbers(array_nums: [-4, -3, -2, -1, 0]))
Sample Output:
true false true
Go to:
PREV : Write a Swift program to test if every 3 that appears in a given array of integers is next to another 3.
NEXT : Write a Swift program to create a new array that is left shifted from a given array of integers. So [11, 15, 13, 10, 45, 20] will be [15, 13, 10, 45, 20, 11]
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?