Swift Basic Programming Exercise: Check if one of the first 4 elements in a given array of integers is a 7
Write a Swift program to check if one of the first 4 elements in a given array of integers is a 7. The length of the array may be less than 4.
Pictorial Presentation:
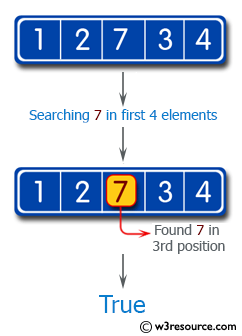
Sample Solution:
Swift Code:
func arrayFront9(_ input: [Int]) -> Bool {
guard input.count > 3 else {
return false
}
if input.prefix(4).contains(7) {
return true
}
return false
}
print(arrayFront9([1, 2, 7, 3, 4]))
print(arrayFront9([1, 2, 3, 4, 7]))
print(arrayFront9([1, 2, 7]))
Sample Output:
true false false
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Swift program to create a Swift program to count the number of 7's in a given array of integers.
Next: Write a Swift program to test if the sequence of numbers 0, 1, 2 appears in a given array of integers somewhere.
What is the difficulty level of this exercise?